Mybatis動態(tài)SQL的實現(xiàn)示例
場景
在實際應用開發(fā)過程中,我們往往需要寫復雜的 SQL 語句,需要拼接,而拼接SQL語句又稍微不注意,由于引號,空格等缺失可能都會導致錯誤。Mybatis提供了動態(tài)SQL,也就是可以根據(jù)用戶提供的參數(shù),動態(tài)決定查詢語句依賴的查詢條件或SQL語句的內容。
動態(tài)SQL標簽
if 和 where 標簽
<!--動態(tài)Sql : where / if--> <select resultType='com.lks.domain.User'> select <include refid='tableAllkey'/> from users <where> <if test='id != null and id != 0'>AND id = #{id} </if> <if test='name != null and name != ’’'>AND name = #{name} </if> <if test='county != null and county != ’’'>AND county = #{county} </if> </where> </select>
一般開發(fā)列表業(yè)務的查詢條件時,如果有多個查詢條件,通常會使用 標簽來進行控制。 標簽可以自動的將第一個條件前面的邏輯運算符 (or ,and) 去掉,正如代碼中寫的,id 查詢條件前面是有“and”關鍵字的,但是在打印出來的 SQL 中卻沒有,這就是 的作用。打印SQL語句的使用可以在mybatis-config文件中添加setting標簽:
<settings> <!-- 打印查詢語句 --> <setting name='logImpl' value='STDOUT_LOGGING' /> </settings>
choose、when、otherwise 標簽
這三個標簽需要組合在一起使用,類似于 Java 中的 switch、case、default。只有一個條件生效,也就是只執(zhí)行滿足的條件 when,沒有滿足的條件就執(zhí)行 otherwise,表示默認條件。
<!--動態(tài)Sql: choose、when、otherwise 標簽--> <select resultType='com.lks.domain.User'> select * from users <where> <choose><when test='name != null and name != ’’'> AND name = #{name}</when><when test='county != null and county != ’’'> AND county = #{county}</when><otherwise> AND id = #{id}</otherwise> </choose> </where> </select>
在測試類中,即使同時添加name和county的值,最終的sql也只會添加第一個屬性值。
set 標簽
使用set標簽可以將動態(tài)的配置 SET 關鍵字,并剔除追加到條件末尾的任何不相關的逗號。使用 if+set 標簽修改后,在進行表單更新的操作中,哪個字段中有值才去更新,如果某項為 null 則不進行更新,而是保持數(shù)據(jù)庫原值。
<!--動態(tài)Sql: set 標簽--> <update parameterType='com.lks.domain.User'> update users <set> <if test='name != null and name != ’’'>name = #{name}, </if> <if test='county != null and county != ’’'>county = #{county}, </if> </set> where id = #{id} </update>
trim 標簽
trim 是一個格式化標簽,可以完成< set > 或者是 < where > 標記的功能。主要有4個參數(shù):① prefix:前綴
② prefixOverrides:去掉第一個and或者是or
③ suffix:后綴
④ suffixOverrides:去掉最后一個逗號,也可以是其他的標記
<!--動態(tài)Sql: trim 標簽--> <select resultType='com.lks.domain.User'> select * from users <trim prefix='where' suffix='order by age' prefixOverrides='and | or' suffixOverrides=','> <if test='name != null and name != ’’'>AND name = #{name} </if> <if test='county != null and county != ’’'>AND county = #{county} </if> </trim> </select>
foreach 標簽
foreach標簽主要有以下參數(shù):item :循環(huán)體中的具體對象。支持屬性的點路徑訪問,如item.age,item.info.details,在list和數(shù)組中是其中的對象,在map中是value。index :在list和數(shù)組中,index是元素的序號,在map中,index是元素的key,該參數(shù)可選。open :表示該語句以什么開始close :表示該語句以什么結束separator :表示元素之間的分隔符,例如在in()的時候,separator=','會自動在元素中間用“,“隔開,避免手動輸入逗號導致sql錯誤,如in(1,2,)這樣。該參數(shù)可選。
list批量插入
<!--動態(tài)Sql: foreach標簽, 批量插入--> <insert useGeneratedKeys='true' keyProperty='id'> insert into users (name, age, county, date) values <foreach collection='list' item='user' separator=',' >(#{user.name}, #{user.age}, #{user.county}, #{user.date}) </foreach> </insert>
從結果可以看出,我們一下插入了兩條數(shù)據(jù),每條數(shù)據(jù)之間使用“,”進行分割,separator=',' 的作用就是如此。其中< foreach >標簽內部的屬性務必加上item.。
list集合參數(shù)
<!--動態(tài)Sql: foreach標簽, list參數(shù)查詢--> <select resultType='com.lks.domain.User'> SELECT * from users WHERE id in <foreach collection='list' item='id' open='(' close=')' separator=',' > #{id} </foreach> </select>
可以看出我們的 SQL 語句新增了:( ? , ? ) ,前后的括號由 open='(' close=')' 進行控制,用“?”占位符占位,并通過separator以:“,”隔開,內部兩個循環(huán)遍歷出的元素。array 集合與 list 的做法也是類似的:
<!--動態(tài)Sql: foreach標簽, array參數(shù)查詢--> <select resultType='com.lks.domain.User'> select * from users WHERE id in <foreach collection='array' item='id' open='(' close=')' separator=','> #{id} </foreach> </select>
map參數(shù)
< map> 標簽需要結合MyBatis的參數(shù)注解 @Param()來使用,需要告訴Mybatis配置文件中的collection='map'里的map是一個參數(shù):
<!--動態(tài)Sql: foreach標簽, map參數(shù)查詢--> <select resultType='com.lks.bean.User'> select * from users WHERE <foreach collection='map' index='key' item='value' separator='='> ${key} = #{value} </foreach> </select>
需要主要${}和#{}的使用。
到此這篇關于Mybatis動態(tài)SQL的實現(xiàn)示例的文章就介紹到這了,更多相關Mybatis動態(tài)SQL內容請搜索好吧啦網(wǎng)以前的文章或繼續(xù)瀏覽下面的相關文章希望大家以后多多支持好吧啦網(wǎng)!
相關文章:
1. Oracle災難防護的關鍵技術2. Microsoft Office Access凍結字段的方法3. 提高商業(yè)智能環(huán)境中DB2查詢的性能(2)4. 關于SQL server中字段值為null的查詢5. 傳甲骨文將增加對MySQL投資與微軟競爭6. 關于Sql server數(shù)據(jù)庫日志滿的快速解決辦法7. Access創(chuàng)建一個簡單MIS管理系統(tǒng)8. SQL Server數(shù)據(jù)庫連接查詢和子查詢實戰(zhàn)案例9. Microsoft Office Access復制數(shù)據(jù)表的方法10. SQL Server靜態(tài)頁面導出技術2
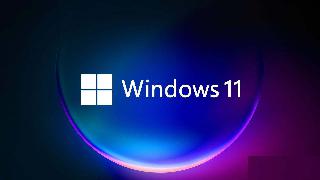