JavaScript 下載鏈接圖片后上傳的實(shí)現(xiàn)
既然要進(jìn)行圖片上傳,那么第一時(shí)間當(dāng)然是判斷是否為可下載的圖片資源,有的時(shí)候可以使用正則表達(dá)式,但是很難判斷是否可下載,當(dāng)判斷圖片鏈接后是否有后綴的時(shí)候也比較苦惱,有的圖片是沒(méi)有后綴的,可是如果放開(kāi)這個(gè)限制又比較容易被攻擊,所以這里我們使用 Image 作為判斷手法,若成功加載圖片,那么說(shuō)明確實(shí)為圖片且可下載。
// 判斷鏈接是否指向圖片且可下載export const checkImgExists = (imgurl: string) => { return new Promise(function (resolve, reject) { var ImgObj = new Image(); ImgObj.src = imgurl; ImgObj.onload = function (res) { resolve(res); }; ImgObj.onerror = function (err) { reject(err); }; });};// how to use itcheckImgExists(imgLink) .then(() => { // do something with imgLink console.log(imgLink); }) .catch((err) => { // some log or alarm console.log(err); console.log('很抱歉, 該鏈接無(wú)法獲取圖片'); });
判斷好后,我們需要對(duì)這個(gè)圖片進(jìn)行下載,這里我們使用 XMLHttpRequest 進(jìn)行請(qǐng)求下載,下載后會(huì)是一個(gè) Blob 對(duì)象。
Blob 本身可以轉(zhuǎn)化成 FormData 對(duì)象或者是 File 對(duì)象,我們可以根據(jù)自己項(xiàng)目的具體情況去選擇上傳策略,如果想傳到 OSS 上,可以選擇轉(zhuǎn)化為 File 對(duì)象,若是傳輸?shù)阶约旱姆?wù)器上,可以使用 Ajax,并轉(zhuǎn) Blob 為 FormData 進(jìn)行上傳。
// 將圖片鏈接中的圖片進(jìn)行 XMLHttpRequest 請(qǐng)求,返回Blob對(duì)象function getImageBlob(url: string) { return new Promise(function (resolve, reject) { var xhr = new XMLHttpRequest(); xhr.open('get', url, true); xhr.responseType = 'blob'; xhr.onload = function () { if (this.status == 200) { resolve(this.response); } }; xhr.onerror = reject; xhr.send(); });}// 將Blob對(duì)象轉(zhuǎn)為File對(duì)象const blobToFile = (blob: Blob, fileName: string) => { return new window.File([blob], fileName, { type: blob.type });};// how to use it// 返回一個(gè)File對(duì)象,可使用該 File 對(duì)象進(jìn)行上傳操作getImageBlob(src).then(async (res: any) => { const srcSplit = src.split('/'); const filename = srcSplit[srcSplit.length - 1]; return blobToFile(res, filename);});
接下來(lái)是一個(gè)上傳OSS的小演示,由于 OSS 涉及的隱私信息較多,所以建議大家把a(bǔ)ccessKeyId、accessKeySecret等信息用接口去獲取,甚至使用臨時(shí)的鑰匙等。
import OSS from 'ali-oss';const ERROR_TIP = '上傳失敗!';/** * File上傳OSS的示例 * 相關(guān)accessKeyId、bucket等參數(shù)需要根據(jù)你的OSS庫(kù)進(jìn)行填寫(xiě) * 建議將【accessKeyId,accessKeySecret】這兩個(gè)敏感信息做成接口獲取或者加密 */export const uploadToOSS = async ( fileName: string, file: File, accessKeyId: string, accessKeySecret: string, ...props) => { let client = new OSS({ endpoint, // 你申請(qǐng)好的oss項(xiàng)目地址 bucket, // OSS 對(duì)象載體 accessKeyId, // your accessKeyId with OSS accessKeySecret, // your accessKeySecret with OSS internal: true, ...props, }); const putResult = await client.put(fileName, file, { timeout: 60 * 1000 * 2, }); if (putResult.res.status === 200) { return { url: putResult.url, fileName }; } throw new Error(ERROR_TIP);};
當(dāng)然如果想上傳圖片到你自己的服務(wù)器,可以選擇將 Blob 格式的文件轉(zhuǎn)為 FormData 格式,使用 XMLHttpRequest 或者 Ajax 進(jìn)行圖片的上傳
// 將Blob對(duì)象轉(zhuǎn)為FormData對(duì)象const blobToFormData = (blob: Blob, fileName: string) => { const formdata = new FormData(); formdata.append('file', blob, fileName); return formdata;};// XMLHttpRequestconst uploadFile = (formData: FormData) => { const url = 'your_interface'; let xhr = new XMLHttpRequest(); xhr.onload = function () { console.log('ok'); console.log(JSON.parse(xhr.responseText)); }; xhr.onerror = function () { console.log('fail'); }; xhr.open('post', url, true); xhr.send(formData);};// Ajaxconst uploadFile2 = (formData: FormData) => { const url = 'your_interface'; $.ajax({ url, type: 'POST', data: formData, async: false, cache: false, contentType: false, processData: false, success: function (returndata) { console.log(returndata); }, error: function (returndata) { console.log(returndata); }, });};
在之前我的后端項(xiàng)目中,使用了 Express 作為靜態(tài)圖片庫(kù),以下是我的 node 上傳圖片代碼。值得注意的是,使用 formidable 解析后,jpg 文件會(huì)直接在你的預(yù)設(shè)照片目錄有一個(gè)很長(zhǎng)的隨機(jī)名稱(chēng),這邊其實(shí)我也是使用了較短的名稱(chēng)進(jìn)行重命名,大家可以根據(jù)自己的需要選擇重命名策略。
const express = require('express');const listenNumber = 5000;const app = express();const bodyParser = require('body-parser');const http = require('http'); //創(chuàng)建服務(wù)器的const formidable = require('formidable');const path = require('path');const fs = require('fs');app.use(express.static('../../upload'));app.use(bodyParser.urlencoded({ extended: false }));app.use(bodyParser.json()); //數(shù)據(jù)JSON類(lèi)型// 上傳圖片app.post('/upLoadArticlePicture', (req, res, next) => { let defaultPath = '../../upload/'; let uploadDir = path.join(__dirname, defaultPath); let form = new formidable.IncomingForm(); let getRandomID = () => Number(Math.random().toString().substr(4, 10) + Date.now()).toString(36); form.uploadDir = uploadDir; //設(shè)置上傳文件的緩存目錄 form.encoding = 'utf-8'; //設(shè)置編輯 form.keepExtensions = true; //保留后綴 form.maxFieldsSize = 2 * 1024 * 1024; //文件大小 form.parse(req, function (err, fields, files) { if (err) { res.locals.error = err; res.render('index', { title: TITLE }); return; } let filePath = files.file['path']; let backName = filePath.split('.')[1]; let oldPath = filePath.split('')[filePath.split('').length - 1]; let newPath = `${getRandomID()}.${backName}`; fs.rename(defaultPath + oldPath, defaultPath + newPath, (err) => { if (!err) { newPath = `http://localhost:${listenNumber}/${newPath}`; res.json({ flag: true, path: newPath }); } else { res.json({ flag: false, path: '' }); } }); });});
到此這篇關(guān)于JavaScript 下載鏈接圖片后上傳的實(shí)現(xiàn)的文章就介紹到這了,更多相關(guān)JavaScript 下載鏈接圖片后上傳內(nèi)容請(qǐng)搜索好吧啦網(wǎng)以前的文章或繼續(xù)瀏覽下面的相關(guān)文章希望大家以后多多支持好吧啦網(wǎng)!
相關(guān)文章:
1. 一個(gè) 2 年 Android 開(kāi)發(fā)者的 18 條忠告2. Vue實(shí)現(xiàn)仿iPhone懸浮球的示例代碼3. js select支持手動(dòng)輸入功能實(shí)現(xiàn)代碼4. vue-drag-chart 拖動(dòng)/縮放圖表組件的實(shí)例代碼5. 什么是Python變量作用域6. Spring的異常重試框架Spring Retry簡(jiǎn)單配置操作7. Android 實(shí)現(xiàn)徹底退出自己APP 并殺掉所有相關(guān)的進(jìn)程8. PHP正則表達(dá)式函數(shù)preg_replace用法實(shí)例分析9. Android studio 解決logcat無(wú)過(guò)濾工具欄的操作10. vue使用moment如何將時(shí)間戳轉(zhuǎn)為標(biāo)準(zhǔn)日期時(shí)間格式
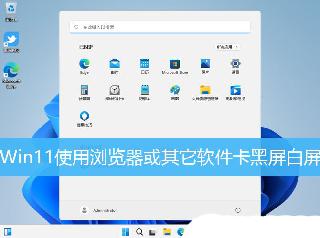