Spring mvc文件上傳下載代碼實例
簡介
文件上傳是項目開發中最常見的功能之一 ,springMVC 可以很好的支持文件上傳,但是SpringMVC上下文中默認沒有裝配MultipartResolver,因此默認情況下其不能處理文件上傳工作。如果想使用Spring的文件上傳功能,則需要在上下文中配置MultipartResolver。
前端表單要求:為了能上傳文件,必須將表單的method設置為POST,并將enctype設置為multipart/form-data。只有在這樣的情況下,瀏覽器才會把用戶選擇的文件以二進制數據發送給服務器;
對表單中的 enctype 屬性做個詳細的說明:
application/x-www=form-urlencoded:默認方式,只處理表單域中的 value 屬性值,采用這種編碼方式的表單會將表單域中的值處理成 URL 編碼方式。
multipart/form-data:這種編碼方式會以二進制流的方式來處理表單數據,這種編碼方式會把文件域指定文件的內容也封裝到請求參數中,不會對字符編碼。
text/plain:除了把空格轉換為 '+' 號外,其他字符都不做編碼處理,這種方式適用直接通過表單發送郵件。
<form action='' enctype='multipart/form-data' method='post'> <input type='file' name='file'/> <input type='submit'></form>
一旦設置了enctype為multipart/form-data,瀏覽器即會采用二進制流的方式來處理表單數據,而對于文件上傳的處理則涉及在服務器端解析原始的HTTP響應。在2003年,Apache Software Foundation發布了開源的Commons FileUpload組件,其很快成為Servlet/JSP程序員上傳文件的最佳選擇。
Servlet3.0規范已經提供方法來處理文件上傳,但這種上傳需要在Servlet中完成。
而Spring MVC則提供了更簡單的封裝。
Spring MVC為文件上傳提供了直接的支持,這種支持是用即插即用的MultipartResolver實現的。
Spring MVC使用Apache Commons FileUpload技術實現了一個MultipartResolver實現類:CommonsMultipartResolver。因此,==SpringMVC的文件上傳還需要依賴Apache Commons FileUpload的組件==。文件上傳
1、導入文件上傳的jar包,commons-fileupload , Maven會自動幫我們導入他的依賴包 commons-io包;
<!--文件上傳--><dependency> <groupId>commons-fileupload</groupId> <artifactId>commons-fileupload</artifactId> <version>1.3.3</version></dependency><!--servlet-api導入高版本的--><dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>4.0.1</version></dependency>
2、配置bean:multipartResolver
【注意!!!這個bena的id必須為:multipartResolver , 否則上傳文件會報400的錯誤!在這里栽過坑,教訓!】
<!--文件上傳配置--><bean class='org.springframework.web.multipart.commons.CommonsMultipartResolver'> <!-- 請求的編碼格式,必須和jSP的pageEncoding屬性一致,以便正確讀取表單的內容,默認為ISO-8859-1 --> <property name='defaultEncoding' value='utf-8'/> <!-- 上傳文件大小上限,單位為字節(10485760=10M) --> <property name='maxUploadSize' value='10485760'/> <property name='maxInMemorySize' value='40960'/></bean>
CommonsMultipartFile 的 常用方法:
String getOriginalFilename():獲取上傳文件的原名 InputStream getInputStream():獲取文件流 void transferTo(File dest):將上傳文件保存到一個目錄文件中我們去實際測試一下
3、編寫前端頁面
<form action='/upload' enctype='multipart/form-data' method='post'> <input type='file' name='file'/> <input type='submit' value='upload'></form>
4、Controller
package com.xiaohua.controller;import org.springframework.stereotype.Controller;import org.springframework.web.bind.annotation.RequestMapping;import org.springframework.web.bind.annotation.RequestParam;import org.springframework.web.multipart.commons.CommonsMultipartFile;import javax.servlet.http.HttpServletRequest;import java.io.*;@Controllerpublic class FileController { //@RequestParam('file') 將name=file控件得到的文件封裝成CommonsMultipartFile 對象 //批量上傳CommonsMultipartFile則為數組即可 @RequestMapping('/upload') public String fileUpload(@RequestParam('file') CommonsMultipartFile file , HttpServletRequest request) throws IOException { //獲取文件名 : file.getOriginalFilename(); String uploadFileName = file.getOriginalFilename(); //如果文件名為空,直接回到首頁! if (''.equals(uploadFileName)){ return 'redirect:/index.jsp'; } System.out.println('上傳文件名 : '+uploadFileName); //上傳路徑保存設置 String path = request.getServletContext().getRealPath('/upload'); //如果路徑不存在,創建一個 File realPath = new File(path); if (!realPath.exists()){ realPath.mkdir(); } System.out.println('上傳文件保存地址:'+realPath); InputStream is = file.getInputStream(); //文件輸入流 OutputStream os = new FileOutputStream(new File(realPath,uploadFileName)); //文件輸出流 //讀取寫出 int len=0; byte[] buffer = new byte[1024]; while ((len=is.read(buffer))!=-1){ os.write(buffer,0,len); os.flush(); } os.close(); is.close(); return 'redirect:/index.jsp'; }}
5、測試上傳文件,OK!
采用file.Transto來保存上傳的文件
編寫Controller
/* * 采用file.Transto 來保存上傳的文件 */@RequestMapping('/upload2')public String fileUpload2(@RequestParam('file') CommonsMultipartFile file, HttpServletRequest request) throws IOException { //上傳路徑保存設置 String path = request.getServletContext().getRealPath('/upload'); File realPath = new File(path); if (!realPath.exists()){ realPath.mkdir(); } //上傳文件地址 System.out.println('上傳文件保存地址:'+realPath); //通過CommonsMultipartFile的方法直接寫文件(注意這個時候) file.transferTo(new File(realPath +'/'+ file.getOriginalFilename())); return 'redirect:/index.jsp';}
前端表單提交地址修改
訪問提交測試
文件下載文件下載步驟:
設置 response 響應頭 讀取文件 -- InputStream 寫出文件 -- OutputStream 執行操作 關閉流 (先開后關)代碼實現:
@RequestMapping(value='/download')public String downloads(HttpServletResponse response ,HttpServletRequest request) throws Exception{ //要下載的圖片地址 String path = request.getServletContext().getRealPath('/upload'); String fileName = '基礎語法.jpg'; //1、設置response 響應頭 response.reset(); //設置頁面不緩存,清空buffer response.setCharacterEncoding('UTF-8'); //字符編碼 response.setContentType('multipart/form-data'); //二進制傳輸數據 //設置響應頭 response.setHeader('Content-Disposition', 'attachment;fileName='+URLEncoder.encode(fileName, 'UTF-8')); File file = new File(path,fileName); //2、 讀取文件--輸入流 InputStream input=new FileInputStream(file); //3、 寫出文件--輸出流 OutputStream out = response.getOutputStream(); byte[] buff =new byte[1024]; int index=0; //4、執行 寫出操作 while((index= input.read(buff))!= -1){ out.write(buff, 0, index); out.flush(); } out.close(); input.close(); return null;}
前端
<a href='http://www.cgvv.com.cn/download' rel='external nofollow' >點擊下載</a>
以上就是本文的全部內容,希望對大家的學習有所幫助,也希望大家多多支持好吧啦網。
相關文章:
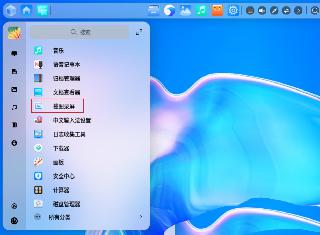