基于spring boot 2和shiro實現身份驗證案例
Shiro是一個功能強大且易于使用的Java安全框架,官網:https://shiro.apache.org/。
主要功能有身份驗證、授權、加密和會話管理。
其它特性有Web支持、緩存、測試支持、允許一個用戶用另一個用戶的身份進行訪問、記住我。
Shiro有三個核心組件:Subject,SecurityManager和 Realm。
Subject:即當前操作“用戶”,“用戶”并不僅僅指人,也可以是第三方進程、后臺帳戶或其他類似事物。
SecurityManager:安全管理器,Shiro框架的核心,通過SecurityManager來管理所有Subject,并通過它來提供安全管理的各種服務。
Realm:域,充當了Shiro與應用安全數據間的“橋梁”或者“連接器”。也就是說,當對用戶執行認證(登錄)和授權(訪問控制)驗證時,Shiro會從應用配置的Realm中查找用戶及其權限信息。當配置Shiro時,必須至少指定一個Realm,用于認證和(或)授權。
Spring Boot 中整合Shiro,根據引入的依賴包shiro-spring和shiro-spring-boot-web-starter(當前版本都是1.4.2)不同有兩種不同方法。
方法一:引入依賴包shiro-spring
1、IDEA中創建一個新的SpringBoot項目,pom.xml引用的依賴包如下:
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.apache.shiro</groupId> <artifactId>shiro-spring</artifactId> <version>1.4.2</version> </dependency>
2、創建Realm和配置shiro
(1)創建Realm
package com.example.demo.config;import org.apache.shiro.authc.*;import org.apache.shiro.authz.AuthorizationInfo;import org.apache.shiro.realm.AuthorizingRealm;import org.apache.shiro.subject.PrincipalCollection;public class MyRealm extends AuthorizingRealm { /**權限信息,暫不實現*/ @Override protected AuthorizationInfo doGetAuthorizationInfo(PrincipalCollection principalCollection) { return null; } /**身份認證:驗證用戶輸入的賬號和密碼是否正確。*/ @Override protected AuthenticationInfo doGetAuthenticationInfo(AuthenticationToken token) throws AuthenticationException { //獲取用戶輸入的賬號 String userName = (String) token.getPrincipal(); //驗證用戶admin和密碼123456是否正確 if (!'admin'.equals(userName)) { throw new UnknownAccountException('賬戶不存在!'); } SimpleAuthenticationInfo authenticationInfo = new SimpleAuthenticationInfo(userName, '123456', getName()); return authenticationInfo; //實際項目中,上面賬號從數據庫中獲取用戶對象,再判斷是否存在 /*User user = userService.findByUserName(userName); if (user == null) { throw new UnknownAccountException('賬戶不存在!'); } SimpleAuthenticationInfo authenticationInfo = new SimpleAuthenticationInfo(user,user.getPassword(), getName()); return authenticationInfo; */ }}
(2)配置Shiro
package com.example.demo.config;import org.apache.shiro.spring.web.ShiroFilterFactoryBean;import org.apache.shiro.web.mgt.DefaultWebSecurityManager;import org.springframework.context.annotation.Bean;import org.springframework.context.annotation.Configuration;import java.util.LinkedHashMap;import java.util.Map;@Configurationpublic class ShiroConfig { @Bean MyRealm myRealm() { return new MyRealm(); } @Bean DefaultWebSecurityManager securityManager() { DefaultWebSecurityManager manager = new DefaultWebSecurityManager(); manager.setRealm(myRealm()); return manager; } @Bean ShiroFilterFactoryBean shiroFilterFactoryBean() { ShiroFilterFactoryBean bean = new ShiroFilterFactoryBean(); bean.setSecurityManager(securityManager()); //如果不設置默認會自動尋找Web工程根目錄下的'/login.jsp'頁面 bean.setLoginUrl('/login'); //登錄成功后要跳轉的鏈接 bean.setSuccessUrl('/index'); //未授權界面 bean.setUnauthorizedUrl('/403'); //配置不會被攔截的鏈接 Map<String, String> map = new LinkedHashMap<>(); map.put('/doLogin', 'anon'); map.put('/**', 'authc'); bean.setFilterChainDefinitionMap(map); return bean; }}
3、控制器測試方法
package com.example.demo.controller;import org.apache.shiro.SecurityUtils;import org.apache.shiro.authc.AuthenticationException;import org.apache.shiro.authc.UnknownAccountException;import org.apache.shiro.authc.UsernamePasswordToken;import org.apache.shiro.subject.Subject;import org.springframework.web.bind.annotation.GetMapping;import org.springframework.web.bind.annotation.PostMapping;import org.springframework.web.bind.annotation.RestController;@RestControllerpublic class LoginController { @GetMapping('/login') public String login() { return '登錄頁面...'; } @PostMapping('/doLogin') public String doLogin(String userName, String password) { Subject subject = SecurityUtils.getSubject(); try { subject.login(new UsernamePasswordToken(userName, password)); return '登錄成功!'; } catch (UnknownAccountException e) { return e.getMessage(); } catch (AuthenticationException e) { return '登陸失敗,密碼錯誤!'; } } //如果沒有先登陸,訪問會跳到/login @GetMapping('/index') public String index() { return 'index'; } @GetMapping('/403') public String unauthorizedRole(){ return '沒有權限'; }}
方法二:引入依賴包shiro-spring-boot-web-starter
1、pom.xml中刪除shiro-spring,引入shiro-spring-boot-web-starter
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.apache.shiro</groupId> <artifactId>shiro-spring-boot-web-starter</artifactId> <version>1.4.2</version> </dependency>
2、創建Realm和配置shiro
(1)創建Realm,代碼和方法一的一樣。
(2)配置Shiro
package com.example.demo.config;import org.apache.shiro.spring.web.config.DefaultShiroFilterChainDefinition;import org.apache.shiro.spring.web.config.ShiroFilterChainDefinition;import org.apache.shiro.web.mgt.DefaultWebSecurityManager;import org.springframework.context.annotation.Bean;import org.springframework.context.annotation.Configuration;@Configurationpublic class ShiroConfig { @Bean MyRealm myRealm() { return new MyRealm(); } @Bean DefaultWebSecurityManager securityManager() { DefaultWebSecurityManager manager = new DefaultWebSecurityManager(); manager.setRealm(myRealm()); return manager; } @Bean ShiroFilterChainDefinition shiroFilterChainDefinition() { DefaultShiroFilterChainDefinition definition = new DefaultShiroFilterChainDefinition(); definition.addPathDefinition('/doLogin', 'anon'); definition.addPathDefinition('/**', 'authc'); return definition; }}
(3)application.yml配置
shiro: unauthorizedUrl: /403 successUrl: /index loginUrl: /login
以上就是本文的全部內容,希望對大家的學習有所幫助,也希望大家多多支持好吧啦網。
相關文章:
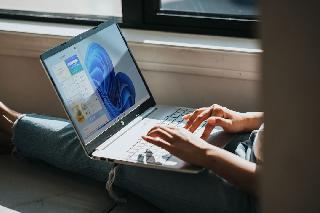