SpringBoot整合Swagger框架過程解析
Swagger 是一個規(guī)范和完整的框架,用于生成、描述、調(diào)用和可視化 RESTful 風格的 Web 服務(wù)。
總體目標是使客戶端和文件系統(tǒng)作為服務(wù)器以同樣的速度來更新。文件的方法、參數(shù)和模型緊密集成到服務(wù)器端的代碼,允許 API 來始終保持同步。Swagger 讓部署管理和使用功能強大的 API 從未如此簡單。
引入maven依賴
<!-- https://mvnrepository.com/artifact/io.springfox/springfox-swagger2 --> <dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger2</artifactId> <version>2.9.2</version> </dependency> <!-- https://mvnrepository.com/artifact/io.springfox/springfox-swagger-ui --> <dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger-ui</artifactId> <version>2.9.2</version> </dependency> <!-- https://mvnrepository.com/artifact/org.projectlombok/lombok --> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <version>1.18.10</version> <scope>provided</scope> </dependency>
創(chuàng)建配置類
package com.example.demo.config;import org.springframework.context.annotation.Bean;import org.springframework.context.annotation.Configuration;import org.springframework.core.env.Environment;import org.springframework.core.env.Profiles;import springfox.documentation.builders.RequestHandlerSelectors;import springfox.documentation.service.ApiInfo;import springfox.documentation.service.Contact;import springfox.documentation.spi.DocumentationType;import springfox.documentation.spring.web.plugins.Docket;import springfox.documentation.swagger2.annotations.EnableSwagger2;import java.util.ArrayList;/** * @author yvioo。 */@Configuration@EnableSwagger2 //開啟Swagger2public class SwaggerConfig { /** * 配置Swagger的Docket的bean實例 * @return */ @Bean public Docket docket(Environment environment) { //設(shè)置只在開發(fā)中環(huán)境中啟動swagger Profiles profiles=Profiles.of('dev'); //表示如果現(xiàn)在是dev環(huán)境,則返回true 開啟swagger boolean flag=environment.acceptsProfiles(profiles); return new Docket(DocumentationType.SWAGGER_2).apiInfo(apiInfo())//是否啟動swagger 默認啟動.enable(flag)//所在分組.groupName('yvioo').select()//指定掃描的包路徑.apis(RequestHandlerSelectors.basePackage('com.example.demo.controller'))//指定掃描的請求,這里表示掃描 /hello/ 的請求//.paths(PathSelectors.ant('/hello/**')).build(); } /** * 配置ApiInfo信息 * @return */ private ApiInfo apiInfo() { //作者信息 Contact author = new Contact('yvioo', 'https://www.cnblogs.com/pxblog/', '[email protected]'); return new ApiInfo('Swagger測試','Swagger描述','1.0','urn:tos',author,'Apache 2.0','http://www.apache.org/licenses/LICENSE-2.0',new ArrayList() ); }}
測試用戶實體類
User.java
package com.example.demo.entity;import io.swagger.annotations.ApiModel;import io.swagger.annotations.ApiModelProperty;import lombok.Data;@ApiModel('用戶實體類 User') //增加實體類接口注釋@Data //使用Lombok插件自動生成get set方法,這樣才能在swagger中顯示屬性值public class User { @ApiModelProperty('用戶ID') //增加字段接口注釋 private Integer id; @ApiModelProperty('用戶名') private String username;}
測試控制器
SwaggerController.java
package com.example.demo.controller;import com.example.demo.entity.User;import io.swagger.annotations.ApiOperation;import io.swagger.annotations.ApiParam;import org.springframework.web.bind.annotation.GetMapping;import org.springframework.web.bind.annotation.PathVariable;import org.springframework.web.bind.annotation.RestController;@RestControllerpublic class SwaggerController { @GetMapping('/hello') public String hello(){ return 'hello'; } /** * 接口返回值含有實體類,就會被swagger掃描 * * @return */ @ApiOperation('查詢用戶方法注釋') @GetMapping(value = '/get/{id}') public User get(@ApiParam('請求參數(shù)注釋') @PathVariable(value = 'id')Integer id){ return new User(); }}
使用dev環(huán)境 啟動項目后 瀏覽器打開http://localhost:8081/swagger-ui.html#/ 我這里用的端口是8081
顯示效果
以上就是本文的全部內(nèi)容,希望對大家的學習有所幫助,也希望大家多多支持好吧啦網(wǎng)。
相關(guān)文章:
1. python 如何在 Matplotlib 中繪制垂直線2. bootstrap select2 動態(tài)從后臺Ajax動態(tài)獲取數(shù)據(jù)的代碼3. ASP常用日期格式化函數(shù) FormatDate()4. python中@contextmanager實例用法5. html中的form不提交(排除)某些input 原創(chuàng)6. CSS3中Transition屬性詳解以及示例分享7. js select支持手動輸入功能實現(xiàn)代碼8. 如何通過python實現(xiàn)IOU計算代碼實例9. 開發(fā)效率翻倍的Web API使用技巧10. vue使用moment如何將時間戳轉(zhuǎn)為標準日期時間格式
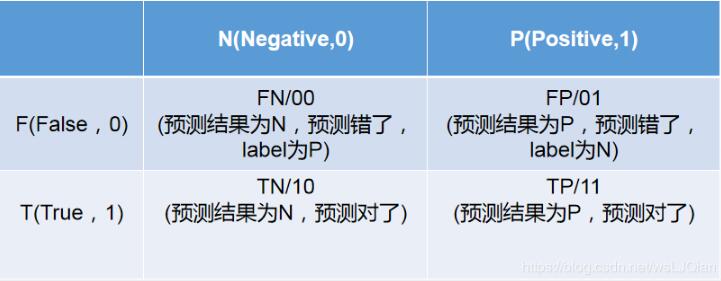