SpringBoot中使用@Scheduled注解創(chuàng)建定時(shí)任務(wù)的實(shí)現(xiàn)
在項(xiàng)目日常開發(fā)過程中,經(jīng)常需要定時(shí)任務(wù)來幫我們做一些工作,如清理日志。定時(shí)任務(wù)的實(shí)現(xiàn)方法主要有 Timer、Quartz 以及 elastic-job
Timer 實(shí)現(xiàn)定時(shí)任務(wù)
只執(zhí)行一次的定時(shí)任務(wù)
Timer timer = new Timer();timer.schedule(new TimerTask() { @Override public void run() { System.out.println('2000毫米后執(zhí)行一次。'); }}, 2000);timer.schedule(new TimerTask() { @Override public void run() { System.out.println('5000毫米后執(zhí)行一次。'); }}, new Date(System.currentTimeMillis() + 5000));
循環(huán)執(zhí)行任務(wù)
Timer timer = new Timer();timer.schedule(new TimerTask() { @Override public void run() { System.out.println(111); }}, 1000, 2000); // 1000毫米后執(zhí)行第一次,之后每2000毫米執(zhí)行一次
終止任務(wù)
timer.concel();
Timer 是 JDK 實(shí)現(xiàn)的定時(shí)任務(wù),用起來簡單、方便,對(duì)一些簡單的定時(shí)任務(wù)可以使用它。由于它不支持 cron 表達(dá)式,現(xiàn)在已經(jīng)很少用了。
Quartz 實(shí)現(xiàn)定時(shí)任務(wù)
Quartz 是一個(gè)完全由 Java 編寫的開源作業(yè)調(diào)度框架,可以用它來實(shí)現(xiàn)定時(shí)任務(wù)。
在 pom.xml 文件添加 Quartz 依賴
<dependency> <groupId>org.quartz-scheduler</groupId> <artifactId>quartz</artifactId> <version>2.2.1</version></dependency><dependency> <groupId>org.quartz-scheduler</groupId> <artifactId>quartz-jobs</artifactId> <version>2.2.1</version></dependency><dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-api</artifactId> <version>1.7.25</version></dependency><dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-simple</artifactId> <version>1.7.6</version></dependency>
編寫 Job
定時(shí)執(zhí)行的任務(wù)
public class QuartzJob implements Job{ public void execute(JobExecutionContext context) throws JobExecutionException { JobDataMap jobDataMap = context.getJobDetail().getJobDataMap(); String hello = (String) jobDataMap.get('hello'); System.err.println(hello); } }
編寫 Task
public void task() { // 該 map 可在 job 中獲取 JobDataMap map = new JobDataMap(); map.put('hello', 'world'); JobDetail jobDetail = newJob(QuartzJob.class). withIdentity('myJob', 'myGroup'). setJobData(map).build(); /* * 簡單定時(shí)器 * * 執(zhí)行時(shí)間間隔 * withIntervalInMilliSeconds 毫秒 * withIntervalInSeconds 秒 * withIntervalInMinutes 分鐘 * withIntervalInHours 小時(shí) * * 執(zhí)行次數(shù) * repeatForever 重復(fù)執(zhí)行 * withRepeatCount 次數(shù) */ SimpleScheduleBuilder scheduleBuilder = simpleSchedule().withIntervalInSeconds(3).withRepeatCount(10); /* * corn定時(shí)器 * * corn表達(dá)式,使用更靈活 * corn表達(dá)式在線生成 http://cron.qqe2.com/ */ CronScheduleBuilder cronScheduleBuilder = CronScheduleBuilder.cronSchedule('0 0 0 1 * ?'); Trigger trigger = newTrigger().startAt(new Date()).//startNow() 默認(rèn)現(xiàn)在開始 withIdentity('myTrigger', 'myGroup'). //withSchedule(scheduleBuilder).build(); withSchedule(cronScheduleBuilder).build(); try { //1.創(chuàng)建Scheduler工廠 SchedulerFactory schedulerFactory = new StdSchedulerFactory(); //2.獲取實(shí)例 Scheduler scheduler = schedulerFactory.getScheduler(); //3.設(shè)置jobDetail詳情和trigger觸發(fā)器 scheduler.scheduleJob(jobDetail, trigger); //4.定時(shí)任務(wù)開始 scheduler.start(); } catch (SchedulerException e) { e.printStackTrace(); }}
在項(xiàng)目啟動(dòng)的時(shí)候調(diào)用 task 方法即可啟動(dòng)定時(shí)任務(wù)。
Spring Boot 創(chuàng)建定時(shí)任務(wù)
Spring Boot 默認(rèn)已經(jīng)實(shí)現(xiàn)了定時(shí)任務(wù),只需要添加相應(yīng)的注解即可完成
pom.xml 文件配置
pom.xml 不需要添加其他依賴,只需要加入 Spring Boot 依賴即可,這里我們添加一個(gè) web 和 test 的依賴
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <optional>true</optional> </dependency></dependencies>
在啟動(dòng)類上面加上 @EnableScheduling 注解
在啟動(dòng)類上面加上 @EnableScheduling 注解即可開啟定時(shí)任務(wù)
@EnableScheduling@SpringBootApplicationpublic class SchedulingApplication { public static void main(String[] args) { SpringApplication.run(SchedulingApplication.class, args); }}
編寫定時(shí)任務(wù)
@Componentpublic class ScheduledTask { @Scheduled(initialDelay=1000, fixedDelay = 1000) public void task1() { System.out.println('延遲1000毫秒后執(zhí)行,任務(wù)執(zhí)行完1000毫秒之后執(zhí)行!'); try { Thread.sleep(2000); } catch (InterruptedException e) { e.printStackTrace(); } } @Scheduled(fixedRate = 2000) public void task2() { System.out.println('延遲1000毫秒后執(zhí)行,之后每2000毫秒執(zhí)行一次!'); }}
除了這些還支持 cron 表達(dá)式
@Scheduled(cron = '*/2 * * * * ?')public void task3() { System.out.println('每2秒執(zhí)行一次!');}
啟動(dòng) Spring Boot 項(xiàng)目在控制臺(tái)就會(huì)看到任務(wù)定時(shí)執(zhí)行
cron 表達(dá)式
以下是 cron 表達(dá)式的的兩種語法
Seconds Minutes Hours DayofMonth Month DayofWeek YearSeconds Minutes Hours DayofMonth Month DayofWeek
每一個(gè)域可出現(xiàn)的字符如下:
Seconds:可出現(xiàn)', - * /'四個(gè)字符,有效范圍為0-59的整數(shù) Minutes:可出現(xiàn)', - * /'四個(gè)字符,有效范圍為0-59的整數(shù) Hours:可出現(xiàn)', - * /'四個(gè)字符,有效范圍為0-23的整數(shù) DayofMonth:可出現(xiàn)', - * / ? L W C'八個(gè)字符,有效范圍為0-31的整數(shù) Month:可出現(xiàn)', - * /'四個(gè)字符,有效范圍為1-12的整數(shù)或JAN-DEc DayofWeek:可出現(xiàn)', - * / ? L C #'四個(gè)字符,有效范圍為1-7的整數(shù)或SUN-SAT兩個(gè)范圍。1表示星期天,2表示星期一, 依次類推 Year:可出現(xiàn)', - * /'四個(gè)字符,有效范圍為1970-2099年舉幾個(gè)例子
*/2 * * * * ? 表示每2秒執(zhí)行一次!0 0 2 1 * ? * 表示在每月的1日的凌晨2點(diǎn)調(diào)度任務(wù) 0 15 10 ? * MON-FRI 表示周一到周五每天上午10:15執(zhí)行作業(yè) 0 15 10 ? 6L 2002-2006 表示2002-2006年的每個(gè)月的最后一個(gè)星期五上午10:15執(zhí)行作
推薦一個(gè) cron 表達(dá)式在線生成工具
http://tools.jb51.net/code/Quartz_Cron_create
參考資料
本文所有代碼放在 Github 上
到此這篇關(guān)于SpringBoot中使用@Scheduled注解創(chuàng)建定時(shí)任務(wù)的實(shí)現(xiàn)的文章就介紹到這了,更多相關(guān)SpringBoot創(chuàng)建定時(shí)任務(wù)內(nèi)容請(qǐng)搜索好吧啦網(wǎng)以前的文章或繼續(xù)瀏覽下面的相關(guān)文章希望大家以后多多支持好吧啦網(wǎng)!
相關(guān)文章:
1. ASP常用日期格式化函數(shù) FormatDate()2. Python 操作 MySQL數(shù)據(jù)庫3. Python數(shù)據(jù)相關(guān)系數(shù)矩陣和熱力圖輕松實(shí)現(xiàn)教程4. 開發(fā)效率翻倍的Web API使用技巧5. bootstrap select2 動(dòng)態(tài)從后臺(tái)Ajax動(dòng)態(tài)獲取數(shù)據(jù)的代碼6. CSS3中Transition屬性詳解以及示例分享7. js select支持手動(dòng)輸入功能實(shí)現(xiàn)代碼8. 什么是Python變量作用域9. vue使用moment如何將時(shí)間戳轉(zhuǎn)為標(biāo)準(zhǔn)日期時(shí)間格式10. python 如何在 Matplotlib 中繪制垂直線
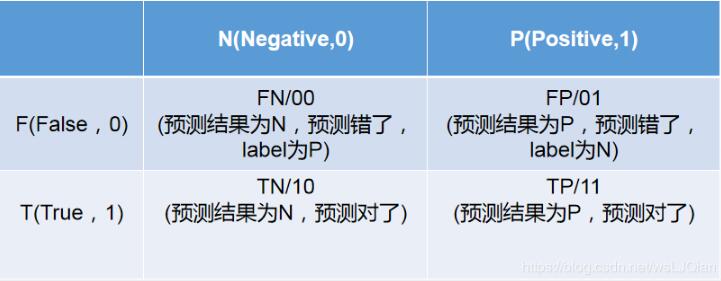