Android使用ExpandableListView實現三層嵌套折疊菜單
前段時間項目的新功能里有些頁面需要三層嵌套列表實現,雖然在移動端這種很丑,但是需求就是需求。本來想用各種View嵌套,然后發現系統有個ExpandableListView。就直接拿來用了。
理論上來說,ExpandableListView的二級嵌套和三級嵌套沒有本質區別,如果把二級嵌套的子級換成一個新的ExpandableListView,就可以實現三級嵌套。
有了思路,關于ExpandableListView的三層嵌套就直接上手實現
這里說下我的需求是有些數據是只有二級,有些數據是三級的。如果你的需求是只有三級,不需要考慮三級二級混合的情況,下面有說明怎么處理。
效果圖
ExpandableListView是官方提供的一個可展示折疊列表的控件。
它的基本用法如下
基本用法ExpandableListView的基本用法很簡單,它本質上就是ListView,所以用法也差不多,這里就不介紹了。
下面開始進入正題。
布局文件先說下,因為是三級嵌套,所以需要四個布局文件,Activity頁面本身需要一個布局文件,然后就是三級嵌套的三個布局文件。
Activity布局文件
<?xml version='1.0' encoding='utf-8'?><LinearLayout xmlns:android='http://schemas.android.com/apk/res/android' android:orientation='vertical' android:layout_width='match_parent' android:layout_height='match_parent'> <ExpandableListView android: android:layout_width='match_parent' android:layout_height='match_parent' android:cacheColorHint='#00000000' android:childIndicator='@color/white' android:divider='@null' android:fadeScrollbars='false' android:groupIndicator='@null' android:listSelector='#00000000' android:scrollbars='none' /></LinearLayout>
我們可以通過ExpandableListView的默認屬性來控制部分樣式,這里貼上菜鳥教程的屬性圖片
一級菜單布局文件
<?xml version='1.0' encoding='utf-8'?><androidx.constraintlayout.widget.ConstraintLayout xmlns:android='http://schemas.android.com/apk/res/android' android:layout_width='match_parent' android:layout_height='44dp' xmlns:app='http://schemas.android.com/apk/res-auto' android:background='@drawable/chapter_gradient_group'> <TextViewandroid: android:layout_width='0dp'android:layout_height='match_parent'app:layout_constraintStart_toStartOf='parent'app:layout_constraintTop_toTopOf='parent'app:layout_constraintBottom_toBottomOf='parent'app:layout_constraintEnd_toEndOf='parent'android:layout_marginHorizontal='10dp'android:paddingStart='20dp'android:singleLine='true'android:ellipsize='end'android:text='@string/groupName'android:textColor='@color/white'android:textSize='16sp'android:gravity='start|center_vertical' /></androidx.constraintlayout.widget.ConstraintLayout>
二級菜單布局文件
<?xml version='1.0' encoding='utf-8'?><androidx.constraintlayout.widget.ConstraintLayout xmlns:android='http://schemas.android.com/apk/res/android' xmlns:app='http://schemas.android.com/apk/res-auto' android:layout_width='match_parent' android:layout_height='match_parent' android:background='@drawable/chapter_gradient_child'> <TextViewandroid: android:layout_width='match_parent'android:layout_height='40dp'android:ellipsize='end'android:gravity='start|center_vertical'android:paddingStart='30dp'android:paddingEnd='10dp'android:singleLine='true'android:text='@string/childName'android:textColor='@color/white'app:layout_constraintEnd_toEndOf='parent'app:layout_constraintStart_toStartOf='parent'app:layout_constraintTop_toTopOf='parent' /></androidx.constraintlayout.widget.ConstraintLayout>
三級菜單布局文件
<?xml version='1.0' encoding='utf-8'?><androidx.constraintlayout.widget.ConstraintLayout xmlns:android='http://schemas.android.com/apk/res/android' xmlns:app='http://schemas.android.com/apk/res-auto' android:layout_width='match_parent' android:layout_height='match_parent' android:background='@drawable/chapter_gradient_grandson'> <TextViewandroid: android:layout_width='match_parent'android:layout_height='40dp'android:ellipsize='end'android:gravity='start|center_vertical'android:paddingStart='40dp'android:paddingEnd='10dp'android:singleLine='true'android:text='@string/grandsonName'app:layout_constraintEnd_toEndOf='parent'app:layout_constraintStart_toStartOf='parent'app:layout_constraintTop_toTopOf='parent' /></androidx.constraintlayout.widget.ConstraintLayout>Adapter
上面說過ExpandableListView繼承自ListView,所以我們需要Adapter,三級嵌套,我們需要兩個Adapter。
這里有必要說一下,為什么是兩個Adapter,ExpandableListView的Adapter繼承自BaseExpandableListAdapter。需要重寫getGroupView和getChildView。這兩個方法中的view分別inflate父級菜單的布局和子級菜單的布局文件。
所以我們上面的三個級別的菜單布局文件通過兩個Adapter來連接。分別是一級菜單的Adapter和三級菜單的Adapter。
下面給出這兩個Adapter的詳細說明,需要注意的地方已經進行備注,請仔細看備注
一級菜單Adapter最值得注意的是該Adapter的getChildView方法和getChildrenCount。因為有些數據不包含三級菜單,有些包含了三級菜單。另外,這個地方需要對下級嵌套的ExpandableListView進行處理。
/** * 三級折疊菜單的一級Adapter * * @author StarryRivers */public class ChapterExpandableAdapter extends BaseExpandableListAdapter {... @Override public int getGroupCount() {// 父菜單長度return fatherChapterList.size(); } @Override public int getChildrenCount(int groupPosition) {// 子菜單長度,嵌套所以返回只能1return 1; } @Override public View getGroupView(int groupPosition, boolean isExpanded, View convertView, ViewGroup parent) {GroupViewHolder groupHolder;// 盡可能重用舊view處理if (convertView == null) { convertView = LayoutInflater.from(parent.getContext()).inflate(R.layout.adapter_expandable_group_view, parent, false); groupHolder = new GroupViewHolder(); groupHolder.groupTitle = convertView.findViewById(R.id.adapter_title); convertView.setTag(groupHolder);} else { groupHolder = (GroupViewHolder) convertView.getTag();}// 設置titlegroupHolder.groupTitle.setText(fatherChapterList.get(groupPosition).getName());return convertView; } @Override public View getChildView(int groupPosition, int childPosition, boolean isLastChild, View convertView, ViewGroup parent) {if (convertView == null) { convertView = new CustomExpandableListView(context);}CustomExpandableListView expandableListView = (CustomExpandableListView) convertView;// 加載子級AdapterChapterExpandableLowAdapter lowAdapter = new ChapterExpandableLowAdapter(context);lowAdapter.setTotalList(fatherChapterList.get(groupPosition).getSec());expandableListView.setAdapter(lowAdapter); if (fatherChapterList.get(groupPosition).getSec().get(childPosition).getThird().size() == 0) { expandableListView.setGroupIndicator(null);}// 本身的父級,相當于三級目錄的子級監聽expandableListView.setOnGroupClickListener((parent12, v, groupPosition12, id) -> { // 如果第三層size為0,意味著沒有三級菜單 if (fatherChapterList != null && fatherChapterList.size() > 0 && fatherChapterList.get(groupPosition).getSec().get(groupPosition12).getThird().size() == 0) {// TODO 業務處理 } // 存在第三級數據,事件分發機制繼續想下傳遞 return false;});expandableListView.setOnChildClickListener((parent1, v, groupPosition1, childPosition1, id) -> { // 三級菜單的業務處理 return true;});return expandableListView; } /** * 子列表是否可選,如果為false,則子項不能觸發點擊事件,默認為false * * @param groupPosition groupPosition * @param childPosition childPosition * @return result */ @Override public boolean isChildSelectable(int groupPosition, int childPosition) {return true; } /** * 父級菜單的ViewHolder */ static class GroupViewHolder {TextView groupTitle; }}
三級菜單Adapter三級菜單的Adapter就和普通的二級嵌套時的Adapter相同,沒什么特別注意的地方,所以只列出了getGroupView和getChildView方法代碼
@Override public View getGroupView(int groupPosition, boolean isExpanded, View convertView, ViewGroup parent) {ChapterExpandableLowAdapter.GroupViewHolder groupHolder;// 盡可能重用舊view處理if (convertView == null) { convertView = LayoutInflater.from(parent.getContext()).inflate(R.layout.adapter_expandable_child_view, parent, false); groupHolder = new ChapterExpandableLowAdapter.GroupViewHolder(); groupHolder.groupTitle = convertView.findViewById(R.id.adapter_child_title); convertView.setTag(groupHolder);} else { groupHolder = (ChapterExpandableLowAdapter.GroupViewHolder) convertView.getTag();}// 設置titlegroupHolder.groupTitle.setText(childChapterList.get(groupPosition).getName());return convertView; } @Override public View getChildView(int groupPosition, int childPosition, boolean isLastChild, View convertView, ViewGroup parent) {ChapterExpandableLowAdapter.ChildViewHolder childHolder;if (convertView == null) { convertView = LayoutInflater.from(parent.getContext()).inflate(R.layout.adapter_expandable_grandson_view, parent, false); childHolder = new ChapterExpandableLowAdapter.ChildViewHolder(); childHolder.childTitle = convertView.findViewById(R.id.adapter_grandson_title); convertView.setTag(childHolder);} else { childHolder = (ChapterExpandableLowAdapter.ChildViewHolder) convertView.getTag();}if (childChapterList.get(groupPosition).getThird() != null && childChapterList.get(groupPosition).getThird().size() > 0) { childHolder.childTitle.setText(childChapterList.get(groupPosition).getThird().get(childPosition).getName());}return convertView; }使用
當我們完成了上面的步驟之后,最后就是在Activity中的使用了。使用方法超級簡單
給ExpandableListView設置Adapter就可以了
@BindView(R.id.chapter_elv) ExpandableListView chapterExpandable; private ChapterExpandableAdapter chapterExpandableAdapter;... chapterExpandableAdapter = new ChapterExpandableAdapter(this); chapterExpandable.setAdapter(chapterExpandableAdapter);寫在最后
因為是三級嵌套,所以ExpandableListView需要重寫一下,重新繪制高度。不然會出現頁面展示不全或者不完整的問題。
以上就是Android使用ExpandableListView實現三層嵌套折疊菜單的詳細內容,更多關于Android ExpandableListView三層嵌套折疊菜單的資料請關注好吧啦網其它相關文章!
相關文章:
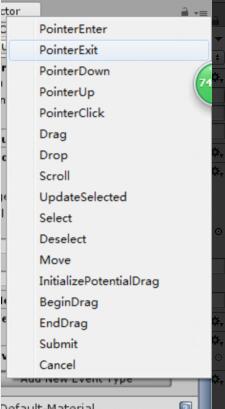