PHP基礎(chǔ)之運算符4——位運算符
位運算符允許對整型數(shù)中指定的位進行求值和操作。
位運算符例子名稱結(jié)果$a & $bAnd(按位與)將把?$a?和?$b?中都為 1 的位設(shè)為 1。$a | $bOr(按位同或)將把?$a?和?$b?中任何一個為 1 的位設(shè)為 1。$a ^ $bXor(按位異或)將把?$a?和?$b?中一個為 1 另一個為 0 的位設(shè)為 1。~ $aNot(按位取反)將?$a?中為 0 的位設(shè)為 1,反之亦然。$a << $bShift left(左移)將?$a?中的位向左移動?$b?次(每一次移動都表示“乘以 2”)。$a >> $bShift right(右移)將?$a?中的位向右移動?$b?次(每一次移動都表示“除以 2”)。位移在 PHP 中是數(shù)學(xué)運算。向任何方向移出去的位都被丟棄。左移時右側(cè)以零填充,符號位被移走意味著正負號不被保留。右移時左側(cè)以符號位填充,意味著正負號被保留。
要用括號確保想要的優(yōu)先級。例如?$a & $b == true?先進行比較再進行按位與;而?($a & $b) == true?則先進行按位與再進行比較。
要注意數(shù)據(jù)類型的轉(zhuǎn)換。如果左右參數(shù)都是字符串,則位運算符將對字符的 ASCII 值進行操作。PHP 的 ini 設(shè)定 error_reporting 使用了按位的值,提供了關(guān)閉某個位的真實例子。要顯示除了提示級別之外的所有錯誤,php.ini 中是這樣用的:E_ALL & ~E_NOTICE
具體運作方式是先取得 E_ALL 的值:00000000000000000111011111111111再取得 E_NOTICE 的值:00000000000000000000000000001000然后通過 ~ 將其取反:11111111111111111111111111110111最后再用按位與 AND(&)得到兩個值中都設(shè)定了(為 1)的位:00000000000000000111011111110111
另外一個方法是用按位異或 XOR(^)來取得只在其中一個值中設(shè)定了的位:E_ALL ^ E_NOTICEerror_reporting 也可用來演示怎樣置位。只顯示錯誤和可恢復(fù)錯誤的方法是:E_ERROR | E_RECOVERABLE_ERROR
也就是將 E_ERROR00000000000000000000000000000001和 E_RECOVERABLE_ERROR00000000000000000001000000000000用按位或 OR(|)運算符來取得在任何一個值中被置位的結(jié)果:00000000000000000001000000000001
Example #1 整數(shù)的 AND,OR 和 XOR 位運算符
<?php/**?Ignore?the?top?section,*?it?is?just?formatting?to?make?output?clearer.*/$format?=?’(%1$2d?=?%1$04b)?=?(%2$2d?=?%2$04b)’.?’?%3$s?(%4$2d?=?%4$04b)’?.?'n';echo?<<<EOH---------?????---------??--?---------result????????value??????op?test---------?????---------??--?---------EOH;/**?Here?are?the?examples.*/$values?=?array(0,?1,?2,?4,?8);$test?=?1?+?4;echo?'n?Bitwise?AND?n';foreach?($values?as?$value)?{$result?=?$value?&?$test;printf($format,?$result,?$value,?’&’,?$test);}echo?'n?Bitwise?Inclusive?OR?n';foreach?($values?as?$value)?{$result?=?$value?|?$test;printf($format,?$result,?$value,?’|’,?$test);}echo?'n?Bitwise?Exclusive?OR?(XOR)?n';foreach?($values?as?$value)?{$result?=?$value?^?$test;printf($format,?$result,?$value,?’^’,?$test);}?>
以上例程會輸出:
--------- --------- -- --------- resultvalue op test --------- --------- -- --------- Bitwise AND( 0 = 0000) = ( 0 = 0000) & ( 5 = 0101)( 1 = 0001) = ( 1 = 0001) & ( 5 = 0101)( 0 = 0000) = ( 2 = 0010) & ( 5 = 0101)( 4 = 0100) = ( 4 = 0100) & ( 5 = 0101)( 0 = 0000) = ( 8 = 1000) & ( 5 = 0101) Bitwise Inclusive OR( 5 = 0101) = ( 0 = 0000) | ( 5 = 0101)( 5 = 0101) = ( 1 = 0001) | ( 5 = 0101)( 7 = 0111) = ( 2 = 0010) | ( 5 = 0101)( 5 = 0101) = ( 4 = 0100) | ( 5 = 0101)(13 = 1101) = ( 8 = 1000) | ( 5 = 0101) Bitwise Exclusive OR (XOR)( 5 = 0101) = ( 0 = 0000) ^ ( 5 = 0101)( 4 = 0100) = ( 1 = 0001) ^ ( 5 = 0101)( 7 = 0111) = ( 2 = 0010) ^ ( 5 = 0101)( 1 = 0001) = ( 4 = 0100) ^ ( 5 = 0101)(13 = 1101) = ( 8 = 1000) ^ ( 5 = 0101)
Example #2 字符串的 XOR 運算符
<?phpecho?12?^?9;?//?Outputs?’5’echo?'12'?^?'9';?//?Outputs?the?Backspace?character?(ascii?8)//?(’1’?(ascii?49))?^?(’9’?(ascii?57))?=?#8echo?'hallo'?^?'hello';?//?Outputs?the?ascii?values?#0?#4?#0?#0?#0//?’a’?^?’e’?=?#4echo?2?^?'3';?//?Outputs?1//?2?^?((int)'3')?==?1echo?'2'?^?3;?//?Outputs?1//?((int)'2')?^?3?==?1?>
Example #3 整數(shù)的位移
<?php/**?Here?are?the?examples.*/echo?'n---?BIT?SHIFT?RIGHT?ON?POSITIVE?INTEGERS?---n';$val?=?4;$places?=?1;$res?=?$val?>>?$places;p($res,?$val,?’>>’,?$places,?’copy?of?sign?bit?shifted?into?left?side’);$val?=?4;$places?=?2;$res?=?$val?>>?$places;p($res,?$val,?’>>’,?$places);$val?=?4;$places?=?3;$res?=?$val?>>?$places;p($res,?$val,?’>>’,?$places,?’bits?shift?out?right?side’);$val?=?4;$places?=?4;$res?=?$val?>>?$places;p($res,?$val,?’>>’,?$places,?’same?result?as?above;?can?not?shift?beyond?0’);echo?'n---?BIT?SHIFT?RIGHT?ON?NEGATIVE?INTEGERS?---n';$val?=?-4;$places?=?1;$res?=?$val?>>?$places;p($res,?$val,?’>>’,?$places,?’copy?of?sign?bit?shifted?into?left?side’);$val?=?-4;$places?=?2;$res?=?$val?>>?$places;p($res,?$val,?’>>’,?$places,?’bits?shift?out?right?side’);$val?=?-4;$places?=?3;$res?=?$val?>>?$places;p($res,?$val,?’>>’,?$places,?’same?result?as?above;?can?not?shift?beyond?-1’);echo?'n---?BIT?SHIFT?LEFT?ON?POSITIVE?INTEGERS?---n';$val?=?4;$places?=?1;$res?=?$val?<<?$places;p($res,?$val,?’<<’,?$places,?’zeros?fill?in?right?side’);$val?=?4;$places?=?(PHP_INT_SIZE?*?8)?-?4;$res?=?$val?<<?$places;p($res,?$val,?’<<’,?$places);$val?=?4;$places?=?(PHP_INT_SIZE?*?8)?-?3;$res?=?$val?<<?$places;p($res,?$val,?’<<’,?$places,?’sign?bits?get?shifted?out’);$val?=?4;$places?=?(PHP_INT_SIZE?*?8)?-?2;$res?=?$val?<<?$places;p($res,?$val,?’<<’,?$places,?’bits?shift?out?left?side’);echo?'n---?BIT?SHIFT?LEFT?ON?NEGATIVE?INTEGERS?---n';$val?=?-4;$places?=?1;$res?=?$val?<<?$places;p($res,?$val,?’<<’,?$places,?’zeros?fill?in?right?side’);$val?=?-4;$places?=?(PHP_INT_SIZE?*?8)?-?3;$res?=?$val?<<?$places;p($res,?$val,?’<<’,?$places);$val?=?-4;$places?=?(PHP_INT_SIZE?*?8)?-?2;$res?=?$val?<<?$places;p($res,?$val,?’<<’,?$places,?’bits?shift?out?left?side,?including?sign?bit’);/**?Ignore?this?bottom?section,*?it?is?just?formatting?to?make?output?clearer.*/function?p($res,?$val,?$op,?$places,?$note?=?’’)?{$format?=?’%0’?.?(PHP_INT_SIZE?*?8)?.?'bn';printf('Expression:?%d?=?%d?%s?%dn',?$res,?$val,?$op,?$places);echo?'?Decimal:n';printf('??val=%dn',?$val);printf('??res=%dn',?$res);echo?'?Binary:n';printf(’??val=’?.?$format,?$val);printf(’??res=’?.?$format,?$res);if?($note)?{echo?'?NOTE:?$noten';}echo?'n';}?>
以上例程在 32 位機器上的輸出:
--- BIT SHIFT RIGHT ON POSITIVE INTEGERS ---Expression: 2 = 4 >> 1 Decimal: val=4 res=2 Binary: val=00000000000000000000000000000100 res=00000000000000000000000000000010 NOTE: copy of sign bit shifted into left sideExpression: 1 = 4 >> 2 Decimal: val=4 res=1 Binary: val=00000000000000000000000000000100 res=00000000000000000000000000000001Expression: 0 = 4 >> 3 Decimal: val=4 res=0 Binary: val=00000000000000000000000000000100 res=00000000000000000000000000000000 NOTE: bits shift out right sideExpression: 0 = 4 >> 4 Decimal: val=4 res=0 Binary: val=00000000000000000000000000000100 res=00000000000000000000000000000000 NOTE: same result as above; can not shift beyond 0--- BIT SHIFT RIGHT ON NEGATIVE INTEGERS ---Expression: -2 = -4 >> 1 Decimal: val=-4 res=-2 Binary: val=11111111111111111111111111111100 res=11111111111111111111111111111110 NOTE: copy of sign bit shifted into left sideExpression: -1 = -4 >> 2 Decimal: val=-4 res=-1 Binary: val=11111111111111111111111111111100 res=11111111111111111111111111111111 NOTE: bits shift out right sideExpression: -1 = -4 >> 3 Decimal: val=-4 res=-1 Binary: val=11111111111111111111111111111100 res=11111111111111111111111111111111 NOTE: same result as above; can not shift beyond -1--- BIT SHIFT LEFT ON POSITIVE INTEGERS ---Expression: 8 = 4 << 1 Decimal: val=4 res=8 Binary: val=00000000000000000000000000000100 res=00000000000000000000000000001000 NOTE: zeros fill in right sideExpression: 1073741824 = 4 << 28 Decimal: val=4 res=1073741824 Binary: val=00000000000000000000000000000100 res=01000000000000000000000000000000Expression: -2147483648 = 4 << 29 Decimal: val=4 res=-2147483648 Binary: val=00000000000000000000000000000100 res=10000000000000000000000000000000 NOTE: sign bits get shifted outExpression: 0 = 4 << 30 Decimal: val=4 res=0 Binary: val=00000000000000000000000000000100 res=00000000000000000000000000000000 NOTE: bits shift out left side--- BIT SHIFT LEFT ON NEGATIVE INTEGERS ---Expression: -8 = -4 << 1 Decimal: val=-4 res=-8 Binary: val=11111111111111111111111111111100 res=11111111111111111111111111111000 NOTE: zeros fill in right sideExpression: -2147483648 = -4 << 29 Decimal: val=-4 res=-2147483648 Binary: val=11111111111111111111111111111100 res=10000000000000000000000000000000Expression: 0 = -4 << 30 Decimal: val=-4 res=0 Binary: val=11111111111111111111111111111100 res=00000000000000000000000000000000 NOTE: bits shift out left side, including sign bit
以上例程在 64 位機器上的輸出:
--- BIT SHIFT RIGHT ON POSITIVE INTEGERS ---Expression: 2 = 4 >> 1 Decimal: val=4 res=2 Binary: val=0000000000000000000000000000000000000000000000000000000000000100 res=0000000000000000000000000000000000000000000000000000000000000010 NOTE: copy of sign bit shifted into left sideExpression: 1 = 4 >> 2 Decimal: val=4 res=1 Binary: val=0000000000000000000000000000000000000000000000000000000000000100 res=0000000000000000000000000000000000000000000000000000000000000001Expression: 0 = 4 >> 3 Decimal: val=4 res=0 Binary: val=0000000000000000000000000000000000000000000000000000000000000100 res=0000000000000000000000000000000000000000000000000000000000000000 NOTE: bits shift out right sideExpression: 0 = 4 >> 4 Decimal: val=4 res=0 Binary: val=0000000000000000000000000000000000000000000000000000000000000100 res=0000000000000000000000000000000000000000000000000000000000000000 NOTE: same result as above; can not shift beyond 0--- BIT SHIFT RIGHT ON NEGATIVE INTEGERS ---Expression: -2 = -4 >> 1 Decimal: val=-4 res=-2 Binary: val=1111111111111111111111111111111111111111111111111111111111111100 res=1111111111111111111111111111111111111111111111111111111111111110 NOTE: copy of sign bit shifted into left sideExpression: -1 = -4 >> 2 Decimal: val=-4 res=-1 Binary: val=1111111111111111111111111111111111111111111111111111111111111100 res=1111111111111111111111111111111111111111111111111111111111111111 NOTE: bits shift out right sideExpression: -1 = -4 >> 3 Decimal: val=-4 res=-1 Binary: val=1111111111111111111111111111111111111111111111111111111111111100 res=1111111111111111111111111111111111111111111111111111111111111111 NOTE: same result as above; can not shift beyond -1--- BIT SHIFT LEFT ON POSITIVE INTEGERS ---Expression: 8 = 4 << 1 Decimal: val=4 res=8 Binary: val=0000000000000000000000000000000000000000000000000000000000000100 res=0000000000000000000000000000000000000000000000000000000000001000 NOTE: zeros fill in right sideExpression: 4611686018427387904 = 4 << 60 Decimal: val=4 res=4611686018427387904 Binary: val=0000000000000000000000000000000000000000000000000000000000000100 res=0100000000000000000000000000000000000000000000000000000000000000Expression: -9223372036854775808 = 4 << 61 Decimal: val=4 res=-9223372036854775808 Binary: val=0000000000000000000000000000000000000000000000000000000000000100 res=1000000000000000000000000000000000000000000000000000000000000000 NOTE: sign bits get shifted outExpression: 0 = 4 << 62 Decimal: val=4 res=0 Binary: val=0000000000000000000000000000000000000000000000000000000000000100 res=0000000000000000000000000000000000000000000000000000000000000000 NOTE: bits shift out left side--- BIT SHIFT LEFT ON NEGATIVE INTEGERS ---Expression: -8 = -4 << 1 Decimal: val=-4 res=-8 Binary: val=1111111111111111111111111111111111111111111111111111111111111100 res=1111111111111111111111111111111111111111111111111111111111111000 NOTE: zeros fill in right sideExpression: -9223372036854775808 = -4 << 61 Decimal: val=-4 res=-9223372036854775808 Binary: val=1111111111111111111111111111111111111111111111111111111111111100 res=1000000000000000000000000000000000000000000000000000000000000000Expression: 0 = -4 << 62 Decimal: val=-4 res=0 Binary: val=1111111111111111111111111111111111111111111111111111111111111100 res=0000000000000000000000000000000000000000000000000000000000000000 NOTE: bits shift out left side, including sign bit
Warning
不要在 32 位系統(tǒng)下向右移超過 32 位。不要在結(jié)果可能超過 32 的情況下左移。使用 gmp 擴展對超出 PHP_INT_MAX 的數(shù)值來進行位操作。
相關(guān)文章:
1. Struts2獲取參數(shù)的三種方法總結(jié)2. JSP中Servlet的Request與Response的用法與區(qū)別3. IntelliJ IDEA刪除類的方法步驟4. js select支持手動輸入功能實現(xiàn)代碼5. Android 實現(xiàn)徹底退出自己APP 并殺掉所有相關(guān)的進程6. vue cli4下環(huán)境變量和模式示例詳解7. vue使用moment如何將時間戳轉(zhuǎn)為標(biāo)準(zhǔn)日期時間格式8. Django視圖類型總結(jié)9. IntelliJ IDEA導(dǎo)入jar包的方法10. Xml簡介_動力節(jié)點Java學(xué)院整理
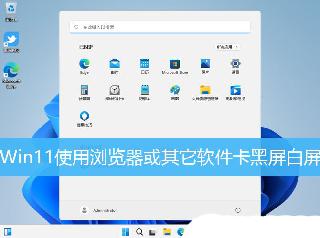