使用java API實現(xiàn)zip遞歸壓縮和解壓文件夾
一、概述
在本篇文章中,給大家介紹一下如何將文件進(jìn)行zip壓縮以及如何對zip包解壓。所有這些都是使用Java提供的核心庫java.util.zip來實現(xiàn)的。
二、壓縮文件
首先我們來學(xué)習(xí)一個簡單的例子-壓縮單個文件。將一個名為test1.txt的文件壓縮到一個名為Compressed.zip的zip文件中。
public class ZipFile { public static void main(String[] args) throws IOException { //輸出壓縮包 FileOutputStream fos = new FileOutputStream('src/main/resources/compressed.zip'); ZipOutputStream zipOut = new ZipOutputStream(fos); //被壓縮文件 File fileToZip = new File('src/main/resources/test1.txt'); FileInputStream fis = new FileInputStream(fileToZip); //向壓縮包中添加文件 ZipEntry zipEntry = new ZipEntry(fileToZip.getName()); zipOut.putNextEntry(zipEntry); byte[] bytes = new byte[1024]; int length; while((length = fis.read(bytes)) >= 0) { zipOut.write(bytes, 0, length); } zipOut.close(); fis.close(); fos.close(); }}
三、壓縮多個文件
接下來,我們看看如何將多個文件壓縮為一個zip文件。我們將把test1.txt和test2.txt壓縮成multiCompressed.zip:
public class ZipMultipleFiles { public static void main(String[] args) throws IOException { List<String> srcFiles = Arrays.asList('src/main/resources/test1.txt', 'src/main/resources/test2.txt'); FileOutputStream fos = new FileOutputStream('src/main/resources/multiCompressed.zip'); ZipOutputStream zipOut = new ZipOutputStream(fos); //向壓縮包中添加多個文件 for (String srcFile : srcFiles) { File fileToZip = new File(srcFile); FileInputStream fis = new FileInputStream(fileToZip); ZipEntry zipEntry = new ZipEntry(fileToZip.getName()); zipOut.putNextEntry(zipEntry); byte[] bytes = new byte[1024]; int length; while((length = fis.read(bytes)) >= 0) { zipOut.write(bytes, 0, length); } fis.close(); } zipOut.close(); fos.close(); }}
四、壓縮目錄
下面的例子,我們將zipTest目錄及該目錄下的遞歸子目錄文件,全都壓縮到dirCompressed.zip中:
public class ZipDirectory { public static void main(String[] args) throws IOException, FileNotFoundException { //被壓縮的文件夾 String sourceFile = 'src/main/resources/zipTest'; //壓縮結(jié)果輸出,即壓縮包 FileOutputStream fos = new FileOutputStream('src/main/resources/dirCompressed.zip'); ZipOutputStream zipOut = new ZipOutputStream(fos); File fileToZip = new File(sourceFile); //遞歸壓縮文件夾 zipFile(fileToZip, fileToZip.getName(), zipOut); //關(guān)閉輸出流 zipOut.close(); fos.close(); } /** * 將fileToZip文件夾及其子目錄文件遞歸壓縮到zip文件中 * @param fileToZip 遞歸當(dāng)前處理對象,可能是文件夾,也可能是文件 * @param fileName fileToZip文件或文件夾名稱 * @param zipOut 壓縮文件輸出流 * @throws IOException */ private static void zipFile(File fileToZip, String fileName, ZipOutputStream zipOut) throws IOException { //不壓縮隱藏文件夾 if (fileToZip.isHidden()) { return; } //判斷壓縮對象如果是一個文件夾 if (fileToZip.isDirectory()) { if (fileName.endsWith('/')) { //如果文件夾是以“/”結(jié)尾,將文件夾作為壓縮箱放入zipOut壓縮輸出流 zipOut.putNextEntry(new ZipEntry(fileName)); zipOut.closeEntry(); } else { //如果文件夾不是以“/”結(jié)尾,將文件夾結(jié)尾加上“/”之后作為壓縮箱放入zipOut壓縮輸出流 zipOut.putNextEntry(new ZipEntry(fileName + '/')); zipOut.closeEntry(); } //遍歷文件夾子目錄,進(jìn)行遞歸的zipFile File[] children = fileToZip.listFiles(); for (File childFile : children) { zipFile(childFile, fileName + '/' + childFile.getName(), zipOut); } //如果當(dāng)前遞歸對象是文件夾,加入ZipEntry之后就返回 return; } //如果當(dāng)前的fileToZip不是一個文件夾,是一個文件,將其以字節(jié)碼形式壓縮到壓縮包里面 FileInputStream fis = new FileInputStream(fileToZip); ZipEntry zipEntry = new ZipEntry(fileName); zipOut.putNextEntry(zipEntry); byte[] bytes = new byte[1024]; int length; while ((length = fis.read(bytes)) >= 0) { zipOut.write(bytes, 0, length); } fis.close(); }} 要壓縮子目錄及其子目錄文件,所以需要遞歸遍歷 每次遍歷找到的是目錄時,我們都將其名稱附加“/”,并將其以ZipEntry保存到壓縮包中,從而保持壓縮的目錄結(jié)構(gòu)。 每次遍歷找到的是文件時,將其以字節(jié)碼形式壓縮到壓縮包里面
五、解壓縮zip壓縮包
下面為大家舉例講解解壓縮zip壓縮包。在此示例中,我們將compressed.zip解壓縮到名為unzipTest的新文件夾中。
public class UnzipFile { public static void main(String[] args) throws IOException { //被解壓的壓縮文件 String fileZip = 'src/main/resources/unzipTest/compressed.zip'; //解壓的目標(biāo)目錄 File destDir = new File('src/main/resources/unzipTest'); byte[] buffer = new byte[1024]; ZipInputStream zis = new ZipInputStream(new FileInputStream(fileZip)); //獲取壓縮包中的entry,并將其解壓 ZipEntry zipEntry = zis.getNextEntry(); while (zipEntry != null) { File newFile = newFile(destDir, zipEntry); FileOutputStream fos = new FileOutputStream(newFile); int len; while ((len = zis.read(buffer)) > 0) { fos.write(buffer, 0, len); } fos.close(); //解壓完成一個entry,再解壓下一個 zipEntry = zis.getNextEntry(); } zis.closeEntry(); zis.close(); } //在解壓目標(biāo)文件夾,新建一個文件 public static File newFile(File destinationDir, ZipEntry zipEntry) throws IOException { File destFile = new File(destinationDir, zipEntry.getName()); String destDirPath = destinationDir.getCanonicalPath(); String destFilePath = destFile.getCanonicalPath(); if (!destFilePath.startsWith(destDirPath + File.separator)) { throw new IOException('該解壓項在目標(biāo)文件夾之外: ' + zipEntry.getName()); } return destFile; }}
總結(jié)
到此這篇關(guān)于使用java API實現(xiàn)zip遞歸壓縮文件夾及解壓的文章就介紹到這了,更多相關(guān)java API實現(xiàn)zip遞歸壓縮文件夾及解壓內(nèi)容請搜索好吧啦網(wǎng)以前的文章或繼續(xù)瀏覽下面的相關(guān)文章希望大家以后多多支持好吧啦網(wǎng)!
相關(guān)文章:
1. js select支持手動輸入功能實現(xiàn)代碼2. 如何在PHP中讀寫文件3. java加載屬性配置properties文件的方法4. PHP正則表達(dá)式函數(shù)preg_replace用法實例分析5. 什么是Python變量作用域6. 《Java程序員修煉之道》作者Ben Evans:保守的設(shè)計思想是Java的最大優(yōu)勢7. CSS3中Transition屬性詳解以及示例分享8. php redis setnx分布式鎖簡單原理解析9. bootstrap select2 動態(tài)從后臺Ajax動態(tài)獲取數(shù)據(jù)的代碼10. vue使用moment如何將時間戳轉(zhuǎn)為標(biāo)準(zhǔn)日期時間格式
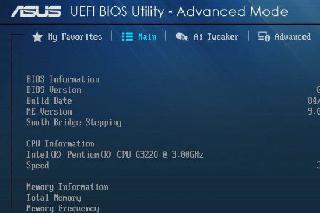