java 將字符串追加到文件已有內容后面的操作
我就廢話不多說了,大家還是直接看代碼吧~
/** * 將字符串追加到文件已有內容后面 * * @param fileFullPath 文件完整地址:D:/test.txt * @param content 需要寫入的 */ public static void writeFile(String fileFullPath,String content) { FileOutputStream fos = null; try { //true不覆蓋已有內容 fos = new FileOutputStream(fileFullPath, true); //寫入 fos.write(content.getBytes()); // 寫入一個換行 fos.write('rn'.getBytes());} catch (IOException e) { e.printStackTrace(); }finally{ if(fos != null){ try { fos.flush(); fos.close(); } catch (IOException e) { e.printStackTrace(); } } } }
補充知識:java寫文件時往末尾追加文件(而不是覆蓋原文件),的兩種方法總結
代碼如下:
import java.io.FileWriter;import java.io.IOException;import java.io.RandomAccessFile; public class AppendToFile { /** * A方法追加文件:使用RandomAccessFile */ public static void appendMethodA(String fileName, String content) { try { // 打開一個隨機訪問文件流,按讀寫方式 RandomAccessFile randomFile = new RandomAccessFile(fileName, 'rw'); // 文件長度,字節數 long fileLength = randomFile.length(); //將寫文件指針移到文件尾。在該位置發生下一個讀取或寫入操作。 randomFile.seek(fileLength); //按字節序列將該字符串寫入該文件。 randomFile.writeBytes(content); //關閉此隨機訪問文件流并釋放與該流關聯的所有系統資源。 randomFile.close(); } catch (IOException e) { e.printStackTrace(); } } /** * B方法追加文件:使用FileWriter */ public static void appendMethodB(String fileName, String content) { try { //打開一個寫文件器,構造函數中的第二個參數true表示以追加形式寫文件,如果為 true,則將字節寫入文件末尾處,而不是寫入文件開始處 FileWriter writer = new FileWriter(fileName, true); writer.write(content); writer.close(); } catch (IOException e) { e.printStackTrace(); } } public static void main(String[] args) { String fileName = 'C:/Temp.txt'; String content = 'new append!'; //按方法A追加文件 AppendToFile.appendMethodA(fileName, content); AppendToFile.appendMethodA(fileName, 'append end. n'); //顯示文件內容 ReadFromFile.readFileByLines(fileName); //按方法B追加文件 AppendToFile.appendMethodB(fileName, content); AppendToFile.appendMethodB(fileName, 'append end. n'); //顯示文件內容 ReadFromFile.readFileByLines(fileName); }}
java控制臺輸出結果如下:
++++++readFileByLines:++++++
以行為單位讀取文件內容,一次讀一整行:
line 1: Sun Yat-sen(November 12, 1866?March 12, 1925) was a Chinese revolutionary and political leader who is often referred to as the 'father of modern China'. Sun played an instrumental and leadership role in the eventual overthrow of the Qing Dynasty in 1911. He was the first provisional president when the Republic of China was founded in 1912. He later co-founded the Kuomintang (KMT) where he served as its first leader. new append!append end.
++++++readFileByLines:++++++
以行為單位讀取文件內容,一次讀一整行:
line 1: Sun Yat-sen(November 12, 1866?March 12, 1925) was a Chinese revolutionary and political leader who is often referred to as the 'father of modern China'. Sun played an instrumental and leadership role in the eventual overthrow of the Qing Dynasty in 1911. He was the first provisional president when the Republic of China was founded in 1912. He later co-founded the Kuomintang (KMT) where he served as its first leader. new append!append end. line 2: new append!append end.
以上這篇java 將字符串追加到文件已有內容后面的操作就是小編分享給大家的全部內容了,希望能給大家一個參考,也希望大家多多支持好吧啦網。
相關文章:
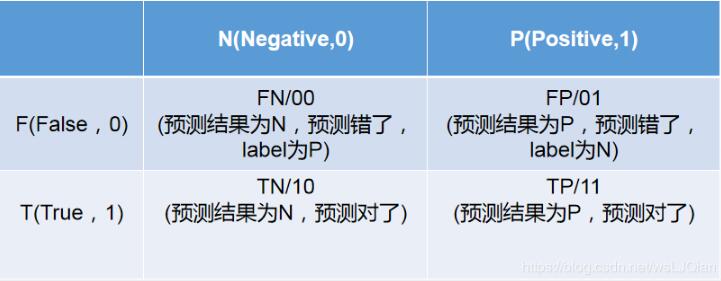