徹底搞懂Java多線程(四)
實(shí)現(xiàn)1000個(gè)線程的時(shí)間格式化
package SimpleDateFormat;import java.text.SimpleDateFormat;import java.util.Date;import java.util.concurrent.LinkedBlockingDeque;import java.util.concurrent.ThreadPoolExecutor;import java.util.concurrent.TimeUnit;/** * user:ypc; * date:2021-06-13; * time: 17:30; */public class SimpleDateFormat1 { private static SimpleDateFormat simpleDateFormat = new SimpleDateFormat('mm:ss'); public static void main(String[] args) {ThreadPoolExecutor threadPoolExecutor = new ThreadPoolExecutor(10,10,100,TimeUnit.MILLISECONDS,new LinkedBlockingDeque<>(1000),new ThreadPoolExecutor.DiscardPolicy());for (int i = 0; i < 1001; i++) { int finalI = i; threadPoolExecutor.submit(new Runnable() {@Overridepublic void run() { Date date = new Date(finalI * 1000); myFormatTime(date);} });}threadPoolExecutor.shutdown(); } private static void myFormatTime(Date date){System.out.println(simpleDateFormat.format(date)); }}
產(chǎn)生了線程不安全的問題👇:
這是因?yàn)椋?/p>
多線程的情況下:
線程1在時(shí)間片用完之后,線程2來setTime()那么線程1的得到了線程2的時(shí)間。
所以可以使用加鎖的操作:
就不會(huì)有重復(fù)的時(shí)間了
但是雖然可以解決線程不安全的問題,但是排隊(duì)等待鎖,性能就會(huì)變得低
所以可以使用局部變量:
也解決了線程不安全的問題:
但是每次也都會(huì)創(chuàng)建新的私有變量
那么有沒有一種方案既可以避免加鎖排隊(duì)執(zhí)行,又不會(huì)每次創(chuàng)建任務(wù)的時(shí)候不會(huì)創(chuàng)建私有的變量呢?
那就是ThreadLocal👇:
ThreadLocalThreadLocal的作用就是讓每一個(gè)線程都擁有自己的變量。
那么選擇鎖還是ThreadLocal?
看創(chuàng)建實(shí)列對(duì)象的復(fù)用率,如果復(fù)用率比較高的話,就使用ThreadLocal。
ThreadLocal的原理類ThreadLocal的主要作用就是將數(shù)據(jù)放到當(dāng)前對(duì)象的Map中,這個(gè)Map時(shí)thread類的實(shí)列變量。類ThreadLocal自己不管理、不存儲(chǔ)任何的數(shù)據(jù),它只是數(shù)據(jù)和Map之間的橋梁。
執(zhí)行的流程:數(shù)據(jù)—>ThreadLocal—>currentThread()—>Map。
執(zhí)行后每個(gè)Map存有自己的數(shù)據(jù),Map中的key中存儲(chǔ)的就是ThreadLocal對(duì)象,value就是存儲(chǔ)的值。每個(gè)Thread的Map值只對(duì)當(dāng)前的線程可見,其它的線程不可以訪問當(dāng)前線程對(duì)象中Map的值。當(dāng)前的線程被銷毀,Map也隨之被銷毀,Map中的數(shù)據(jù)如果沒有被引用、沒有被使用,則隨時(shí)GC回收。
ThreadLocal常用方法set(T):將內(nèi)容存儲(chǔ)到ThreadLocal
get():從線程去私有的變量
remove():從線程中移除私有變量
package ThreadLocalDemo;import java.text.SimpleDateFormat;/** * user:ypc; * date:2021-06-13; * time: 18:37; */public class ThreadLocalDemo1 { private static ThreadLocal<SimpleDateFormat> threadLocal = new ThreadLocal<>(); public static void main(String[] args) {//設(shè)置私有變量threadLocal.set(new SimpleDateFormat('mm:ss'));//得到ThreadLocalSimpleDateFormat simpleDateFormat = threadLocal.get();//移除threadLocal.remove(); }}ThreadLocal的初始化
ThreadLocal提供了兩種初始化的方法
initialValue()和
initialValue()初始化:
package ThreadLocalDemo;import java.text.SimpleDateFormat;import java.util.Date;/** * user:ypc; * date:2021-06-13; * time: 19:07; */public class ThreadLocalDemo2 { //創(chuàng)建并初始化ThreadLocal private static ThreadLocal<SimpleDateFormat> threadLocal = new ThreadLocal() {@Overrideprotected SimpleDateFormat initialValue() { System.out.println(Thread.currentThread().getName() + '執(zhí)行了自己的threadLocal中的初始化方法initialValue()'); return new SimpleDateFormat('mm:ss');} }; public static void main(String[] args) {Thread thread1 = new Thread(() -> { Date date = new Date(5000); System.out.println('thread0格式化時(shí)間之后得結(jié)果時(shí):' + threadLocal.get().format(date));});thread1.setName('thread0');thread1.start();Thread thread2 = new Thread(() -> { Date date = new Date(6000); System.out.println('thread1格式化時(shí)間之后得結(jié)果時(shí):' + threadLocal.get().format(date));});thread2.setName('thread1');thread2.start(); }}
withInitial方法初始化:
package ThreadLocalDemo;import java.util.function.Supplier;/** * user:ypc; * date:2021-06-14; * time: 17:23; */public class ThreadLocalDemo3 { private static ThreadLocal<String> stringThreadLocal = ThreadLocal.withInitial(new Supplier<String>() {@Overridepublic String get() { System.out.println('執(zhí)行了withInitial()方法'); return '我是' + Thread.currentThread().getName() + '的ThreadLocal';} }); public static void main(String[] args) {Thread thread1 = new Thread(() -> { System.out.println(stringThreadLocal.get());});thread1.start();Thread thread2 = new Thread(new Runnable() { @Override public void run() {System.out.println(stringThreadLocal.get()); }});thread2.start(); }}
注意:
ThreadLocal如果使用了set()方法的話,那么它的初始化方法就不會(huì)起作用了。
來看:👇
package ThreadLocalDemo;/** * user:ypc; * date:2021-06-14; * time: 18:43; */class Tools { public static ThreadLocal t1 = new ThreadLocal();}class ThreadA extends Thread { @Override public void run() {for (int i = 0; i < 10; i++) { System.out.println('在ThreadA中取值:' + Tools.t1.get()); try {Thread.sleep(100); } catch (InterruptedException e) {e.printStackTrace(); }} }}public class ThreadLocalDemo4 { public static void main(String[] args) throws InterruptedException {//main是ThreadA 的 父線程 讓main線程set,ThreadA,是get不到的if (Tools.t1.get() == null) { Tools.t1.set('main父線程的set');}System.out.println('main get 到了: ' + Tools.t1.get());Thread.sleep(1000);ThreadA a = new ThreadA();a.start(); }}
類ThreadLocal不能實(shí)現(xiàn)值的繼承,那么就可以使用InheritableThreadLocal了👇
InheritableThreadLocal的使用使用InheritableThreadLocal可以使子線程繼承父線程的值
在來看運(yùn)行的結(jié)果:
子線程有最新的值,父線程依舊是舊的值
package ThreadLocalDemo;/** * user:ypc; * date:2021-06-14; * time: 19:07; */class ThreadB extends Thread{ @Override public void run() {for (int i = 0; i < 10; i++) { System.out.println('在ThreadB中取值:' + Tools.t1.get()); if (i == 5){Tools.t1.set('我是ThreadB中新set()'); } try {Thread.sleep(100); } catch (InterruptedException e) {e.printStackTrace(); }} }}public class ThreadLocalDemo5 { public static void main(String[] args) throws InterruptedException {if (Tools.t1.get() == null) { Tools.t1.set('main父線程的set');}System.out.println('main get 到了: ' + Tools.t1.get());Thread.sleep(1000);ThreadA a = new ThreadA();a.start();Thread.sleep(5000);for (int i = 0; i < 10; i++) { System.out.println('main的get是:' + Tools.t1.get()); Thread.sleep(100);} }}
ThreadLocal的臟讀問題來看👇
package ThreadLocalDemo;import java.util.concurrent.LinkedBlockingDeque;import java.util.concurrent.ThreadPoolExecutor;import java.util.concurrent.TimeUnit;/** * user:ypc; * date:2021-06-14; * time: 19:49; */public class ThreadLocalDemo6 { private static ThreadLocal<String> threadLocal = new ThreadLocal<>(); private static class MyThread extends Thread {private static boolean flag = false;@Overridepublic void run() { String name = this.getName(); if (!flag) {threadLocal.set(name);System.out.println(name + '設(shè)置了' + name);flag = true; } System.out.println(name + '得到了' + threadLocal.get());} } public static void main(String[] args) {ThreadPoolExecutor threadPoolExecutor = new ThreadPoolExecutor(1, 1, 0,TimeUnit.MILLISECONDS, new LinkedBlockingDeque<>(10));for (int i = 0; i < 2; i++) { threadPoolExecutor.execute(new MyThread());}threadPoolExecutor.shutdown(); }}
發(fā)生了臟讀:
線程池復(fù)用了線程,也復(fù)用了這個(gè)線程相關(guān)的靜態(tài)屬性,就導(dǎo)致了臟讀
那么如何避免臟讀呢?
去掉static 之后:
本篇文章就到這里了,希望對(duì)你有些幫助,也希望你可以多多關(guān)注好吧啦網(wǎng)的更多內(nèi)容!
相關(guān)文章:
1. js select支持手動(dòng)輸入功能實(shí)現(xiàn)代碼2. PHP橋接模式Bridge Pattern的優(yōu)點(diǎn)與實(shí)現(xiàn)過程3. asp.net core項(xiàng)目授權(quán)流程詳解4. html中的form不提交(排除)某些input 原創(chuàng)5. CSS3中Transition屬性詳解以及示例分享6. bootstrap select2 動(dòng)態(tài)從后臺(tái)Ajax動(dòng)態(tài)獲取數(shù)據(jù)的代碼7. vue使用moment如何將時(shí)間戳轉(zhuǎn)為標(biāo)準(zhǔn)日期時(shí)間格式8. 開發(fā)效率翻倍的Web API使用技巧9. jsp文件下載功能實(shí)現(xiàn)代碼10. ASP常用日期格式化函數(shù) FormatDate()
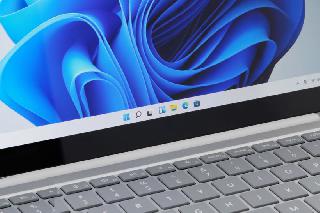