Laravel Eloquent ORM高級(jí)部分解析
目錄
- 查詢(xún)作用域
- 全局作用域
- 本地作用域
- 事件
- 使用場(chǎng)景
- 序列化
- 轉(zhuǎn)換模型/集合為數(shù)組 - toArray()
- 轉(zhuǎn)換模型為json - toJson()
- 隱藏屬性
- 為json追加值
- Mutators
- Accessors & Mutators
- accessors
- mutators
- 屬性轉(zhuǎn)換
查詢(xún)作用域
全局作用域
全局作用域允許你對(duì)給定模型的所有查詢(xún)添加約束。使用全局作用域功能可以為模型的所有操作增加約束。
軟刪除功能實(shí)際上就是利用了全局作用域功能
實(shí)現(xiàn)一個(gè)全局作用域功能只需要定義一個(gè)實(shí)現(xiàn)Illuminate\Database\Eloquent\Scope
接口的類(lèi),該接口只有一個(gè)方法apply
,在該方法中增加查詢(xún)需要的約束
<?php namespace App\Scopes; use Illuminate\Database\Eloquent\Scope; use Illuminate\Database\Eloquent\Model; use Illuminate\Database\Eloquent\Builder; class AgeScope implements Scope { /** * Apply the scope to a given Eloquent query builder. * * @param \Illuminate\Database\Eloquent\Builder $builder * @param \Illuminate\Database\Eloquent\Model $model * @return void */ public function apply(Builder $builder, Model $model) { return $builder->where("age", ">", 200); } }
在模型的中,需要覆蓋其boot
方法,在該方法中增加addGlobalScope
<?php namespace App; use App\Scopes\AgeScope; use Illuminate\Database\Eloquent\Model; class User extends Model { /** * The "booting" method of the model. * * @return void */ protected static function boot() { parent::boot(); static::addGlobalScope(new AgeScope); } }
添加全局作用域之后,User::all()
操作將會(huì)產(chǎn)生如下等價(jià)sql
select * from `users` where `age` > 200
也可以使用匿名函數(shù)添加全局約束
static::addGlobalScope("age", function(Builder $builder) { $builder->where("age", ">", 200); });
查詢(xún)中要移除全局約束的限制,使用withoutGlobalScope
方法
// 只移除age約束 User::withoutGlobalScope("age")->get(); User::withoutGlobalScope(AgeScope::class)->get(); // 移除所有約束 User::withoutGlobalScopes()->get(); // 移除多個(gè)約束 User::withoutGlobalScopes([FirstScope::class, SecondScope::class])->get();
本地作用域
本地作用域只對(duì)部分查詢(xún)添加約束,需要手動(dòng)指定是否添加約束,在模型中添加約束方法,使用前綴scope
<?php namespace App; use Illuminate\Database\Eloquent\Model; class User extends Model { /** * Scope a query to only include popular users. * * @return \Illuminate\Database\Eloquent\Builder */ public function scopePopular($query) { return $query->where("votes", ">", 100); } /** * Scope a query to only include active users. * * @return \Illuminate\Database\Eloquent\Builder */ public function scopeActive($query) { return $query->where("active", 1); } }
使用上述添加的本地約束查詢(xún),只需要在查詢(xún)中使用scope
前綴的方法,去掉scope
前綴即可
$users = App\User::popular()->active()->orderBy("created_at")->get(); // 本地作用域方法是可以接受參數(shù)的 public function scopeOfType($query, $type) { return $query->where("type", $type); } // 調(diào)用的時(shí)候 $users = App\User::ofType("admin")->get();
事件
Eloquent模型會(huì)觸發(fā)下列事件
creating`, `created`, `updating`, `updated`, `saving`, `saved`,`deleting`, `deleted`, `restoring`, `restored
使用場(chǎng)景
假設(shè)我們希望保存用戶(hù)的時(shí)候?qū)τ脩?hù)進(jìn)行校驗(yàn),校驗(yàn)通過(guò)后才允許保存到數(shù)據(jù)庫(kù),可以在服務(wù)提供者中為模型的事件綁定監(jiān)聽(tīng)
<?php namespace App\Providers; use App\User; use Illuminate\Support\ServiceProvider; class AppServiceProvider extends ServiceProvider { /** * Bootstrap any application services. * * @return void */ public function boot() { User::creating(function ($user) { if ( ! $user->isValid()) { return false; } }); } /** * Register the service provider. * * @return void */ public function register() { // } }
上述服務(wù)提供者對(duì)象中,在框架啟動(dòng)時(shí)會(huì)監(jiān)聽(tīng)模型的creating
事件,當(dāng)保存用戶(hù)之間檢查用戶(hù)數(shù)據(jù)的合法性,如果不合法,返回false
,模型數(shù)據(jù)不會(huì)被持久化到數(shù)據(jù)。
返回false會(huì)阻止模型的save
/ update
操作
序列化
當(dāng)構(gòu)建JSON API
的時(shí)候,經(jīng)常會(huì)需要轉(zhuǎn)換模型和關(guān)系為數(shù)組或者json
。Eloquent
提供了一些方法可以方便的來(lái)實(shí)現(xiàn)數(shù)據(jù)類(lèi)型之間的轉(zhuǎn)換。
轉(zhuǎn)換模型/集合為數(shù)組 - toArray()
$user = App\User::with("roles")->first(); return $user->toArray(); $users = App\User::all(); return $users->toArray();
轉(zhuǎn)換模型為json - toJson()
$user = App\User::find(1); return $user->toJson(); $user = App\User::find(1); return (string) $user;
隱藏屬性
有時(shí)某些字段不應(yīng)該被序列化,比如用戶(hù)的密碼等,使用$hidden
字段控制那些字段不應(yīng)該被序列化
<?php namespace App; use Illuminate\Database\Eloquent\Model; class User extends Model { /** * The attributes that should be hidden for arrays. * * @var array */ protected $hidden = ["password"]; }
隱藏關(guān)聯(lián)關(guān)系的時(shí)候,使用的是它的方法名稱(chēng),不是動(dòng)態(tài)的屬性名
也可以使用$visible
指定會(huì)被序列化的白名單
<?php namespace App; use Illuminate\Database\Eloquent\Model; class User extends Model { /** * The attributes that should be visible in arrays. * * @var array */ protected $visible = ["first_name", "last_name"]; } // 有時(shí)可能需要某個(gè)隱藏字段被臨時(shí)序列化,使用`makeVisible`方法 return $user->makeVisible("attribute")->toArray();
為json追加值
有時(shí)需要在json
中追加一些數(shù)據(jù)庫(kù)中不存在的字段,使用下列方法,現(xiàn)在模型中增加一個(gè)get
方法
<?php namespace App; use Illuminate\Database\Eloquent\Model; class User extends Model { /** * The accessors to append to the model"s array form. * * @var array */ protected $appends = ["is_admin"]; /** * Get the administrator flag for the user. * * @return bool */ public function getIsAdminAttribute() { return $this->attributes["admin"] == "yes"; } }
方法簽名為getXXXAttribute
格式,然后為模型的$appends
字段設(shè)置字段名。
Mutators
在Eloquent
模型中,Accessor
和Mutator
可以用來(lái)對(duì)模型的屬性進(jìn)行處理,比如我們希望存儲(chǔ)到表中的密碼字段要經(jīng)過(guò)加密才行,我們可以使用Laravel
的加密工具自動(dòng)的對(duì)它進(jìn)行加密。
Accessors & Mutators
accessors
要定義一個(gè)accessor
,需要在模型中創(chuàng)建一個(gè)名稱(chēng)為getXxxAttribute
的方法,其中的Xxx是駝峰命名法的字段名。
假設(shè)我們有一個(gè)字段是first_name
,當(dāng)我們嘗試去獲取first_name的值的時(shí)候,getFirstNameAttribute
方法將會(huì)被自動(dòng)的調(diào)用
<?php namespace App; use Illuminate\Database\Eloquent\Model; class User extends Model { /** * Get the user"s first name. * * @param string $value * @return string */ public function getFirstNameAttribute($value) { return ucfirst($value); } } // 在訪(fǎng)問(wèn)的時(shí)候,只需要正常的訪(fǎng)問(wèn)屬性就可以 $user = App\User::find(1); $firstName = $user->first_name;
mutators
創(chuàng)建mutators
與accessorsl
類(lèi)似,創(chuàng)建名為setXxxAttribute
的方法即可
<?php namespace App; use Illuminate\Database\Eloquent\Model; class User extends Model { /** * Set the user"s first name. * * @param string $value * @return string */ public function setFirstNameAttribute($value) { $this->attributes["first_name"] = strtolower($value); } } // 賦值方式 $user = App\User::find(1); $user->first_name = "Sally";
屬性轉(zhuǎn)換
模型的$casts
屬性提供了一種非常簡(jiǎn)便的方式轉(zhuǎn)換屬性為常見(jiàn)的數(shù)據(jù)類(lèi)型,在模型中,使用$casts
屬性定義一個(gè)數(shù)組,該數(shù)組的key為要轉(zhuǎn)換的屬性名稱(chēng),value為轉(zhuǎn)換的數(shù)據(jù)類(lèi)型,當(dāng)前支持integer
, real
, float
, double
, string
, boolean
, object
, array
,collection
, date
, datetime
, 和 timestamp
。
<?php namespace App; use Illuminate\Database\Eloquent\Model; class User extends Model { /** * The attributes that should be casted to native types. * * @var array */ protected $casts = [ "is_admin" => "boolean", ]; }
數(shù)組類(lèi)型的轉(zhuǎn)換時(shí)非常有用的,我們?cè)跀?shù)據(jù)庫(kù)中存儲(chǔ)json
數(shù)據(jù)的時(shí)候,可以將其轉(zhuǎn)換為數(shù)組形式。
<?php namespace App; use Illuminate\Database\Eloquent\Model; class User extends Model { /** * The attributes that should be casted to native types. * * @var array */ protected $casts = [ "options" => "array", ]; } // 從配置數(shù)組轉(zhuǎn)換的屬性取值或者賦值的時(shí)候都會(huì)自動(dòng)的完成json和array的轉(zhuǎn)換 $user = App\User::find(1); $options = $user->options; $options["key"] = "value"; $user->options = $options; $user->save();
以上就是Laravel Eloquent ORM高級(jí)部分解析的詳細(xì)內(nèi)容,更多關(guān)于Laravel Eloquent ORM解析的資料請(qǐng)關(guān)注其它相關(guān)文章!
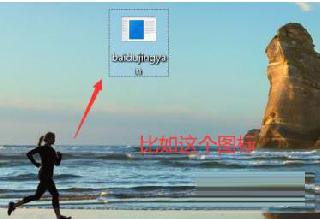