python 字符串格式化的示例
一、舊式的字符串格式化
% 操作符
參考以下示例:
>>> name = 'Eric'>>> 'Hello, %s.' % name’Hello, Eric.’
當有多個變量需要插入到字符串中時:
>>> name = 'Eric'>>> age = 74>>> 'Hello, %s. You are %s.' % (name, age)’Hello, Eric. You are 74.’
當需要替換的變量進一步增多時,使用 % 操作符格式化字符串會導致代碼可讀性變得很差:
>>> first_name = 'Eric'>>> last_name = 'Idle'>>> age = 74>>> profession = 'comedian'>>> affiliation = 'Monty Python'>>> 'Hello, %s %s. You are %s. You are a %s. You were a member of %s.' % (first_name, last_name, age, profession, affiliation)’Hello, Eric Idle. You are 74. You are a comedian. You were a member of Monty Python.’
str.format()
str.format() 是對 % 方式的改進,它使用常見的函數調用的語法,并且可以通過定義對象本身的 __format__() 方法控制字符串格式化的具體行為。
基本用法:
>>> name = 'Eric'>>> age = 74>>> 'Hello, {}. You are {}.'.format(name, age)’Hello, Eric. You are 74.’
str.format() 相對于 % 操作符有著更強的靈活性。比如可以通過數字索引來關聯替換到字符串中的變量:
>>> name = 'Eric'>>> age = 74>>> 'Hello, {1}. You are {0}.'.format(age, name)’Hello, Eric. You are 74.’
為了提高代碼可讀性,{} 中也可以使用有具體含義的參數名:
>>> name = 'Eric'>>> age = 74>>> 'Hello, {name}. You are {age}'.format(name=name, age=age)’Hello, Eric. You are 74’
針對字典結構的數據:
>>> person = {’name’: ’Eric’, ’age’: 74}>>> 'Hello, {name}. You are {age}.'.format(name=person[’name’], age=person[’age’])’Hello, Eric. You are 74.’
或者更簡潔的方式:
>>> person = {’name’: ’Eric’, ’age’: 74}>>> 'Hello, {name}. You are {age}.'.format(**person)’Hello, Eric. You are 74.’
問題在于當需要替換的變量很多時,str.format() 方式依然會導致代碼變得過于冗長:
>>> first_name = 'Eric'>>> last_name = 'Idle'>>> age = 74>>> profession = 'comedian'>>> affiliation = 'Monty Python'>>> 'Hello, {first_name} {last_name}. You are {age}. You are a {profession}. You were a member of {affiliation}.' .format(first_name=first_name, last_name=last_name, age=age, profession=profession, affiliation=affiliation)’Hello, Eric Idle. You are 74. You are a comedian. You were a member of Monty Python.’
二、f-string
基本用法
>>> name = 'Eric'>>> age = 74>>> f'Hello, {name}. You are {age}.'’Hello, Eric. You are 74.’
嵌入表達式
>>> f'{2 * 37}'’74’>>> def to_lowercase(input):... return input.lower() >>> name = 'Eric Idle'>>> f'{to_lowercase(name)} is funny'’eric idle is funny’>>> f'{name.lower()} is funny'’eric idle is funny’
f-string 中還可以直接嵌入某個對象實例,只要其內部實現了 __str__ 或者 __repr__ 方法:
class Comedian: def __init__(self, first_name, last_name, age): self.first_name = first_name self.last_name = last_name self.age = age def __str__(self): return f'{self.first_name} {self.last_name} is {self.age}'new_comedian = Comedian('Eric', 'Idle', 74)print(f'{new_comedian}')# Eric Idle is 74
多行 f-string
>>> name = 'Eric'>>> profession = 'comedian'>>> affiliation = 'Monty Python'>>> message = (... f'Hi {name}. '... f'You are a {profession}. '... f'You were in {affiliation}.'... )>>> message’Hi Eric. You are a comedian. You were in Monty Python.’
參考資料
Python 3’s f-Strings: An Improved String Formatting Syntax (Guide)
以上就是python 字符串格式化的示例的詳細內容,更多關于python 字符串格式化的資料請關注好吧啦網其它相關文章!
相關文章:
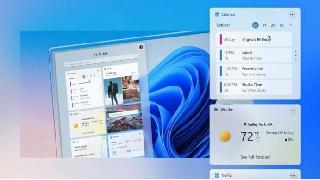